用C语言编写程序从命令行中同时获取输入文件名和输出文件名。
时间: 2024-05-10 17:21:26 浏览: 19
```
#include <stdio.h>
int main(void) {
char input_file[100], output_file[100];
printf("Enter input file name: ");
scanf("%s", input_file);
printf("Enter output file name: ");
scanf("%s", output_file);
printf("Input file name: %s\n", input_file);
printf("Output file name: %s\n", output_file);
return 0;
}
```
注:需要注意的是,如果文件名中含有空格等特殊字符,需要使用双引号将文件名括起来,例如:`./a.out "input file.txt" "output file.txt"`。
相关问题
用c语言编写一个文件拷贝程序,该程序通过命令行中获取原始文件名和目标文件名
以下是一个基本的文件拷贝程序,它通过命令行参数获取原始文件名和目标文件名并将文件内容从原始文件复制到目标文件中。
```c
#include <stdio.h>
int main(int argc, char *argv[]) {
FILE *src_file, *dst_file;
char c;
if (argc != 3) {
printf("Usage: %s <src_file> <dst_file>\n", argv[0]);
return 1;
}
src_file = fopen(argv[1], "rb");
if (src_file == NULL) {
printf("Error opening source file.\n");
return 2;
}
dst_file = fopen(argv[2], "wb");
if (dst_file == NULL) {
printf("Error opening destination file.\n");
fclose(src_file);
return 3;
}
while ((c = fgetc(src_file)) != EOF) {
fputc(c, dst_file);
}
fclose(src_file);
fclose(dst_file);
printf("File copied successfully.\n");
return 0;
}
```
在命令行中执行以下命令来运行该程序:
```
./filecopy input.txt output.txt
```
其中`input.txt`是原始文件名,`output.txt`是目标文件名。程序将从`input.txt`中读取内容并将其写入`output.txt`。如果文件无法打开或读取失败,则程序会在控制台输出错误消息并退出。如果文件成功复制,则程序将输出`File copied successfully.`。
如何用c语言编写命令行程序
要编写命令行程序,你需要遵循以下步骤:
1. 决定程序的功能和参数。
2. 编写代码并指定程序的参数。你可以使用命令行参数,也可以通过标准输入流来获取用户输入。
3. 编译代码并生成可执行文件。使用gcc编译器可以将源代码编译成可执行文件。例如:
```
gcc -o myprog myprog.c
```
其中,myprog为可执行文件名,myprog.c为源代码文件名。
4. 在命令行中运行程序。在终端输入可执行文件名和参数,例如:
```
./myprog arg1 arg2
```
其中,./表示当前目录,myprog为可执行文件名,arg1和arg2为命令行参数。
下面是一个简单的例子,展示如何在C语言中编写一个简单的命令行程序:
```c
#include <stdio.h>
int main(int argc, char *argv[]) {
printf("Hello, world!\n");
if (argc > 1) {
printf("You entered %d command-line arguments:\n", argc - 1);
for (int i = 1; i < argc; i++) {
printf("%s\n", argv[i]);
}
} else {
printf("You did not enter any command-line arguments.\n");
}
return 0;
}
```
这个程序输出“Hello, world!”,然后检查是否有命令行参数,并将它们输出到终端。你可以使用类似以下命令来编译和运行这个程序:
```
gcc -o myprog myprog.c
./myprog arg1 arg2
```
相关推荐
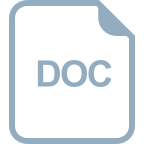
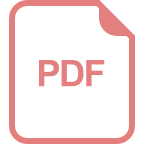
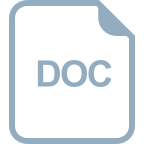












