要求用户可以自己输入,而不是已知的数据
时间: 2024-03-23 13:43:43 浏览: 51
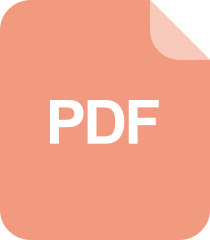
如何用数据看用户行为,驱动产品设计
好的,以下是修改后的代码示例,可以让用户自己输入链表结点的数据:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表结构体
struct Node {
int no; // 学号
int score; // 成绩
struct Node* next;
};
// 定义函数用于在链表中插入结点
void insertNode(struct Node** head, int no, int score) {
struct Node* newNode = (struct Node*) malloc(sizeof(struct Node));
newNode->no = no;
newNode->score = score;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
struct Node* cur = *head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
// 定义函数用于合并两个链表,并按学号升序排序
struct Node* mergeList(struct Node* a, struct Node* b) {
struct Node* result = NULL;
// 如果其中一个链表为空,则直接返回另外一个链表
if (a == NULL) {
return b;
} else if (b == NULL) {
return a;
}
// 比较两个链表的第一个结点的学号大小,将小的结点加入结果链表中
if (a->no <= b->no) {
result = a;
result->next = mergeList(a->next, b);
} else {
result = b;
result->next = mergeList(a, b->next);
}
return result;
}
// 定义函数用于打印链表
void printList(struct Node* head) {
struct Node* cur = head;
while (cur != NULL) {
printf("学号:%d,成绩:%d\n", cur->no, cur->score);
cur = cur->next;
}
}
int main() {
struct Node* a = NULL;
struct Node* b = NULL;
int n, no, score;
// 输入链表a的结点数据
printf("请输入链表a的结点个数:");
scanf("%d", &n);
printf("请依次输入链表a的结点的学号和成绩:\n");
for (int i = 0; i < n; i++) {
scanf("%d %d", &no, &score);
insertNode(&a, no, score);
}
// 输入链表b的结点数据
printf("请输入链表b的结点个数:");
scanf("%d", &n);
printf("请依次输入链表b的结点的学号和成绩:\n");
for (int i = 0; i < n; i++) {
scanf("%d %d", &no, &score);
insertNode(&b, no, score);
}
// 合并链表a和链表b,并按学号升序排序
struct Node* merged = mergeList(a, b);
// 打印结果链表
printf("合并后的链表为:\n");
printList(merged);
return 0;
}
```
这段代码中,在 `main` 函数中,我们先让用户输入链表 `a` 和链表 `b` 的结点个数,然后依次输入每个结点的学号和成绩,最后调用 `mergeList` 函数将它们合并,并按学号升序排序。最后调用 `printList` 函数打印出结果链表。
阅读全文
相关推荐
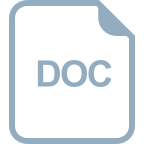
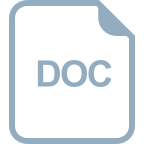
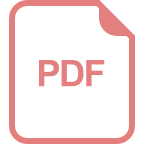
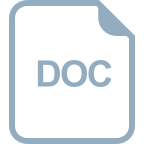
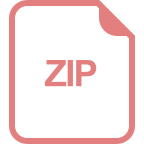
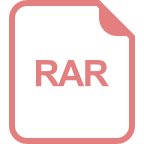
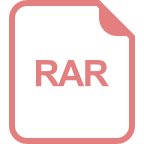
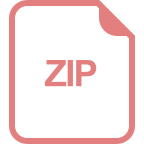
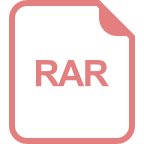
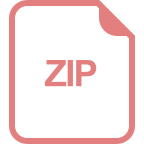
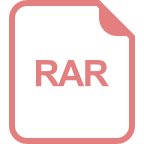
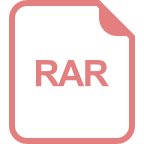
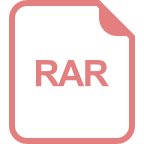
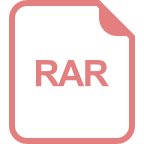
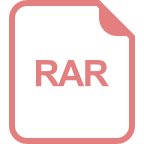
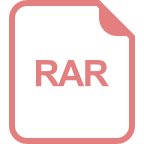
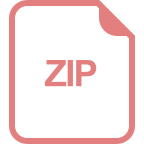
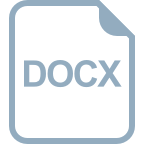