unity代码实现MVC架构框架
时间: 2023-09-21 09:11:29 浏览: 52
Unity 中实现 MVC 架构通常需要以下几步:
1. 创建 Model 类:Model 类用于存储数据和数据操作方法,例如:
```
public class PlayerModel {
private int _health;
public int Health {
get { return _health; }
set {
_health = value;
if (_health <= 0) {
// 触发死亡事件
OnDeath();
}
}
}
public event Action OnDeath;
public void TakeDamage(int amount) {
Health -= amount;
}
}
```
2. 创建 View 类:View 类用于显示数据,例如:
```
public class PlayerView : MonoBehaviour {
public TextMeshProUGUI healthText;
public void UpdateHealth(int health) {
healthText.text = "Health: " + health;
}
}
```
3. 创建 Controller 类:Controller 类用于控制 Model 和 View,例如:
```
public class PlayerController {
private PlayerModel _model;
private PlayerView _view;
public PlayerController(PlayerModel model, PlayerView view) {
_model = model;
_view = view;
// 监听 Model 的数据变化并更新 View
_model.OnHealthChanged += _view.UpdateHealth;
// 监听 View 的用户操作并控制 Model
_view.OnTakeDamage += _model.TakeDamage;
}
}
```
4. 在场景中创建 View 对象,并在 Controller 中传入 Model 和 View:
```
public class GameController : MonoBehaviour {
public PlayerView playerViewPrefab;
private void Start() {
// 创建 Model 对象
var playerModel = new PlayerModel();
// 创建 View 对象并绑定到场景中的 GameObject 上
var playerView = Instantiate(playerViewPrefab);
playerView.transform.SetParent(transform, false);
// 创建 Controller 对象并传入 Model 和 View
var playerController = new PlayerController(playerModel, playerView);
}
}
```
这样就完成了一个简单的 MVC 架构的实现。在实际项目中,可能需要更复杂的 Model 和 View 类,以及更多的 Controller 类来控制不同的数据和视图。
相关推荐
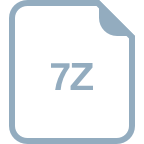














