续上
时间: 2024-02-11 07:09:11 浏览: 73
完整代码如下:
```c
#include <stdio.h>
#include <seal/seal.h>
int main() {
// 创建加密上下文和密钥
seal::EncryptionParameters parms(seal::scheme_type::BFV);
parms.set_poly_modulus_degree(4096);
parms.set_coeff_modulus(seal::CoeffModulus::BFVDefault(4096));
parms.set_plain_modulus(1 << 8);
seal::SEALContext context(parms);
seal::SecretKey secret_key = seal::SecretKey::Create(context);
// 创建加密器和解密器
seal::Encryptor encryptor(context, secret_key);
seal::Decryptor decryptor(context, secret_key);
// 读取txt文件中的数据
FILE *fp;
char line[256];
fp = fopen("location.txt", "r");
if (fp == NULL) {
printf("Failed to open location.txt\n");
return -1;
}
// 加密数据并保存到容器中
std::vector<seal::Ciphertext> encrypted_data;
while (fgets(line, sizeof(line), fp)) {
double longitude, latitude;
sscanf(line, "%lf,%lf", &longitude, &latitude);
seal::Plaintext plaintext;
seal::encode(longitude, context.plain_modulus(), plaintext);
seal::Ciphertext ciphertext;
encryptor.encrypt(plaintext, ciphertext);
encrypted_data.push_back(ciphertext);
}
// 写入加密后的数据到txt文件
fp = fopen("encrypted_location.txt", "w");
if (fp == NULL) {
printf("Failed to open encrypted_location.txt\n");
return -1;
}
for (const auto &encrypted : encrypted_data) {
seal::Plaintext plaintext;
decryptor.decrypt(encrypted, plaintext);
std::string str;
seal::to_string(plaintext, str);
fwrite(str.c_str(), 1, str.length(), fp);
fwrite("\n", 1, 1, fp);
}
fclose(fp);
return 0;
}
```
需要注意的是,SEAL库中的数据类型和API都是C++的,如果需要在C代码中使用SEAL库,需要使用C++编译器将C代码和SEAL库链接起来。可以使用extern "C"来将C++代码中的函数声明为C函数,以便在C代码中调用。
阅读全文
相关推荐
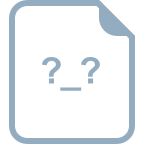
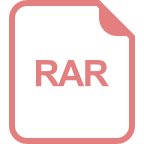
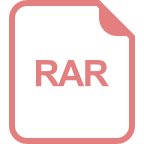
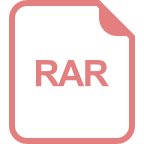
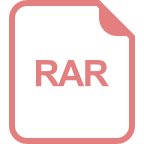
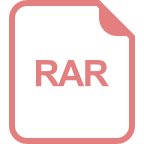
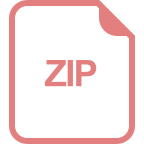
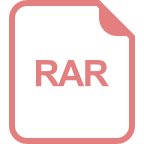
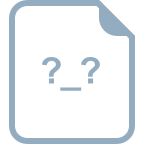
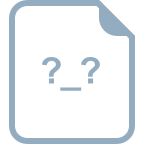
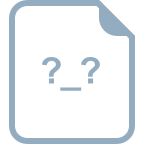
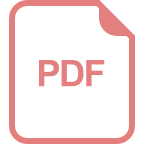
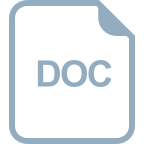
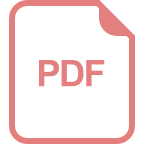