java成语接龙窗口程序 eclipse 具体代码
时间: 2024-03-18 19:42:03 浏览: 31
好的,下面是一个Java成语接龙窗口程序的具体代码,基于Eclipse开发环境:
```java
import java.awt.EventQueue;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.border.EmptyBorder;
public class ChengYuJieLong extends JFrame {
private static final long serialVersionUID = 1L;
private JPanel contentPane;
private JTextField textField;
private JLabel lblNewLabel;
private JLabel lblNewLabel_1;
private JButton btnNewButton;
private JLabel lblNewLabel_2;
private List<String> idiomList = new ArrayList<String>(); // 成语列表
private String currentIdiom; // 当前成语
/**
* Launch the application.
*/
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
ChengYuJieLong frame = new ChengYuJieLong();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
/**
* Create the frame.
*/
public ChengYuJieLong() {
// 初始化成语列表
initIdiomList();
// 随机选择当前成语
currentIdiom = getRandomIdiom();
// 初始化窗口
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 300);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
lblNewLabel = new JLabel("请输入一个成语:");
lblNewLabel.setBounds(10, 10, 150, 30);
lblNewLabel.setFont(new Font("宋体", Font.PLAIN, 16));
contentPane.add(lblNewLabel);
textField = new JTextField();
textField.setBounds(160, 10, 200, 30);
textField.setFont(new Font("宋体", Font.PLAIN, 16));
contentPane.add(textField);
textField.setColumns(10);
lblNewLabel_1 = new JLabel("当前成语:");
lblNewLabel_1.setBounds(10, 50, 150, 30);
lblNewLabel_1.setFont(new Font("宋体", Font.PLAIN, 16));
contentPane.add(lblNewLabel_1);
lblNewLabel_2 = new JLabel(currentIdiom);
lblNewLabel_2.setBounds(160, 50, 200, 30);
lblNewLabel_2.setFont(new Font("宋体", Font.PLAIN, 16));
contentPane.add(lblNewLabel_2);
btnNewButton = new JButton("提交");
btnNewButton.setBounds(160, 90, 100, 30);
btnNewButton.setFont(new Font("宋体", Font.PLAIN, 16));
btnNewButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
String inputIdiom = textField.getText().trim();
if (inputIdiom.isEmpty()) {
JOptionPane.showMessageDialog(null, "成语不能为空!");
} else if (!isValidIdiom(inputIdiom)) {
JOptionPane.showMessageDialog(null, "不是有效的成语!");
} else if (!isValidNextIdiom(inputIdiom)) {
JOptionPane.showMessageDialog(null, "不符合成语接龙规则!");
} else {
// 成功接龙,更新当前成语
currentIdiom = inputIdiom;
lblNewLabel_2.setText(currentIdiom);
// 清空输入框
textField.setText("");
// 随机回答一个成语
String answerIdiom = getRandomIdiom();
JOptionPane.showMessageDialog(null, "回答:" + answerIdiom);
currentIdiom = answerIdiom;
lblNewLabel_2.setText(currentIdiom);
}
}
});
contentPane.add(btnNewButton);
}
/**
* 初始化成语列表
*/
private void initIdiomList() {
// TODO: 这里可以从文件或数据库中读取成语列表
idiomList.add("千军万马");
idiomList.add("万无一失");
idiomList.add("一寸光阴一寸金");
idiomList.add("金玉满堂");
idiomList.add("堂而皇之");
idiomList.add("之乎者也");
idiomList.add("也就是说");
idiomList.add("说曹操,曹操就到");
idiomList.add("到此一游");
idiomList.add("游戏人间");
}
/**
* 判断是否为有效的成语
*/
private boolean isValidIdiom(String idiom) {
// TODO: 这里可以使用字典或API进行判断
return idiomList.contains(idiom);
}
/**
* 判断是否符合成语接龙规则
*/
private boolean isValidNextIdiom(String idiom) {
char lastChar = currentIdiom.charAt(currentIdiom.length() - 1);
char firstChar = idiom.charAt(0);
return lastChar == firstChar;
}
/**
* 随机选择一个成语
*/
private String getRandomIdiom() {
Random rand = new Random();
int index = rand.nextInt(idiomList.size());
return idiomList.get(index);
}
}
```
这个程序中,我们使用了Java Swing库来实现GUI界面,实现了一个简单的成语接龙游戏。需要注意的是,这个程序只是一个示例,功能比较简单,还有很多地方可以进行优化和改进。
相关推荐
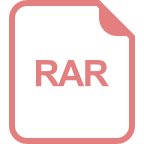
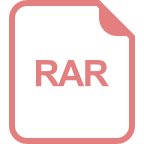














