用java传入长和宽,生成一个迷宫
时间: 2023-06-23 07:10:30 浏览: 44
生成迷宫可以使用随机深度优先搜索算法(Randomized Depth-First Search Algorithm)或者递归分割算法(Recursive Division Algorithm)。下面是一个简单的随机深度优先搜索算法的Java实现,你可以根据自己的需求进行修改。
```java
import java.util.Random;
public class MazeGenerator {
private int width;
private int height;
private int[][] maze;
private Random random;
public MazeGenerator(int width, int height) {
this.width = width;
this.height = height;
maze = new int[height][width];
random = new Random();
}
public void generate() {
// Initialize the maze with all walls
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
maze[i][j] = 1;
}
}
// Choose a random starting point
int startX = random.nextInt(width);
int startY = random.nextInt(height);
maze[startY][startX] = 0;
// Use recursive depth-first search to carve out the maze
dfs(startX, startY);
}
private void dfs(int x, int y) {
int[] directions = {-1, 0, 1, 0, -1};
int[] randomDirections = shuffleArray(directions);
for (int i = 0; i < 4; i++) {
int dx = randomDirections[i];
int dy = randomDirections[i + 1];
int nextX = x + dx;
int nextY = y + dy;
if (nextX >= 0 && nextX < width && nextY >= 0 && nextY < height && maze[nextY][nextX] == 1) {
maze[y + dy / 2][x + dx / 2] = 0;
maze[nextY][nextX] = 0;
dfs(nextX, nextY);
}
}
}
private int[] shuffleArray(int[] array) {
int[] shuffledArray = array.clone();
for (int i = shuffledArray.length - 1; i > 0; i--) {
int j = random.nextInt(i + 1);
int temp = shuffledArray[i];
shuffledArray[i] = shuffledArray[j];
shuffledArray[j] = temp;
}
return shuffledArray;
}
public void print() {
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (maze[i][j] == 1) {
System.out.print("#");
} else {
System.out.print(" ");
}
}
System.out.println();
}
}
public static void main(String[] args) {
MazeGenerator generator = new MazeGenerator(20, 10);
generator.generate();
generator.print();
}
}
```
这个实现使用二维数组来表示迷宫,0代表通路,1代表墙壁。首先将所有格子都初始化为1,然后随机选择一个起点开始搜索,使用递归深度优先搜索算法生成迷宫。在搜索过程中,每次随机打乱四个方向的顺序,然后依次尝试向四个方向移动。如果下一个格子是未访问过的墙壁,就打通当前格子和下一个格子之间的墙壁,然后递归处理下一个格子。最终得到的迷宫可以通过调用print()方法打印出来。
相关推荐
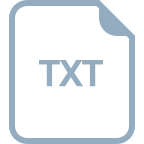
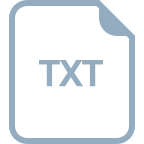














