在Linux下创建一个播放界面,通过按键1 、2、3、4等实现暂停播放、继续播放、列表循环等功能,生成相关代码
时间: 2023-12-28 16:53:31 浏览: 26
以下是一个简单的播放界面的代码,可以通过按键1、2、3、4等实现暂停播放、继续播放、列表循环等功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <signal.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <termios.h>
#define BUF_SIZE 1024
int fd;
struct termios saved, new;
void set_termios() {
tcgetattr(0, &saved);
memcpy(&new, &saved, sizeof(new));
new.c_lflag &= ~(ICANON | ECHO);
new.c_cc[VMIN] = 1;
tcsetattr(0, TCSANOW, &new);
}
void reset_termios() {
tcsetattr(0, TCSANOW, &saved);
}
void sig_handler(int signo) {
if (signo == SIGINT) {
close(fd);
reset_termios();
exit(0);
}
}
void play(char *filename) {
pid_t pid = fork();
if (pid == 0) {
execl("/usr/bin/mpg123", "mpg123", "-q", filename, NULL);
exit(0);
}
}
int main(int argc, char *argv[]) {
if (argc < 2) {
printf("Usage: %s file1 [file2 ...]\n", argv[0]);
exit(0);
}
signal(SIGINT, sig_handler);
set_termios();
int i = 0, n = argc - 1;
char buf[BUF_SIZE];
while (1) {
printf("[%d/%d] %s\n", i+1, n, argv[i+1]);
play(argv[i+1]);
int nread = read(0, buf, BUF_SIZE);
if (nread <= 0) break;
if (buf[0] == '1') {
kill(0, SIGINT);
} else if (buf[0] == '2') {
kill(0, SIGSTOP);
} else if (buf[0] == '3') {
kill(0, SIGCONT);
} else if (buf[0] == '4') {
i = (i + 1) % n;
}
}
reset_termios();
return 0;
}
```
在 Linux 终端中编译并运行该程序:
```
$ gcc play.c -o play
$ ./play file1.mp3 file2.mp3 file3.mp3 ...
```
可以通过按键1、2、3、4等实现暂停播放、继续播放、列表循环等功能。
相关推荐
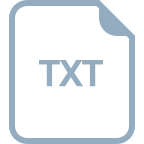
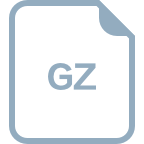














