写一个python通过telnet连接交换机代码
时间: 2023-05-14 12:04:14 浏览: 229
以下是一个简单的 Python 代码示例,用于通过 Telnet 连接到交换机:
```python
import telnetlib
HOST = "192.168.1.1" # 交换机的 IP 地址
user = "username" # 登录用户名
password = "password" # 登录密码
tn = telnetlib.Telnet(HOST)
tn.read_until(b"Username: ")
tn.write(user.encode('ascii') + b"\n")
tn.read_until(b"Password: ")
tn.write(password.encode('ascii') + b"\n")
tn.write(b"enable\n")
tn.write(b"password\n") # enable 密码
tn.write(b"terminal length 0\n") # 设置终端长度为 0,以便一次性显示所有输出
tn.write(b"show interfaces\n") # 执行 show 命令
output = tn.read_all().decode('ascii')
print(output)
tn.close()
```
这个代码示例使用了 Python 的 telnetlib 库,通过 Telnet 协议连接到交换机,并执行了 show interfaces 命令,最后将输出打印到控制台上。
相关问题
写一个python用类的方式通过telnet连接交换机代码
以下是一个简单的 Python 类,用于通过 Telnet 连接到交换机并执行命令:
```python
import telnetlib
class SwitchTelnet:
def __init__(self, host, username, password):
self.host = host
self.username = username
self.password = password
self.tn = None
def connect(self):
self.tn = telnetlib.Telnet(self.host)
self.tn.read_until(b"Username: ")
self.tn.write(self.username.encode('ascii') + b"\n")
self.tn.read_until(b"Password: ")
self.tn.write(self.password.encode('ascii') + b"\n")
def execute(self, command):
self.tn.write(command.encode('ascii') + b"\n")
output = self.tn.read_until(b"#").decode('ascii')
return output
```
使用示例:
```python
switch = SwitchTelnet("192.168.1.1", "admin", "password")
switch.connect()
output = switch.execute("show interfaces")
print(output)
```
请注意,这只是一个简单的示例,实际上您需要根据您的交换机类型和配置进行更改。
写一个Python Telnet远程连接交换机的程序
抱歉,作为AI语言模型,我无法提供Python Telnet远程连接交换机的程序。不过,我可以提供一些参考代码,帮助你开始编写这个程序。
import telnetlib
# 定义交换机的IP地址和端口号
HOST = "192.168.1.1"
PORT = 23
# 定义用户名和密码
user = "admin"
password = "admin123"
# 创建Telnet对象并连接到交换机
tn = telnetlib.Telnet(HOST, PORT)
# 登录交换机
tn.read_until(b"Username: ")
tn.write(user.encode('ascii') + b"\n")
tn.read_until(b"Password: ")
tn.write(password.encode('ascii') + b"\n")
# 执行一些交换机命令
tn.write(b"enable\n")
tn.write(b"show interfaces\n")
tn.write(b"exit\n")
# 读取交换机输出并打印
print(tn.read_all().decode('ascii'))
# 关闭Telnet连接
tn.close()
阅读全文
相关推荐
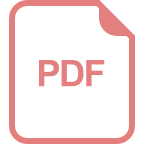









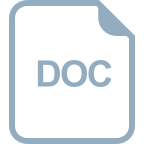



