使用python3编写一段求图像连通区域的代码,要求精度要高
时间: 2024-03-06 16:52:10 浏览: 18
好的,我可以为您提供一段使用Python3实现的图像连通区域的代码,使用的是深度优先搜索算法(DFS)。
首先,需要导入以下模块:
```python
import numpy as np
import cv2
```
然后,读取图像并将其转换为灰度图像:
```python
img = cv2.imread('image.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
接下来,定义一个函数来实现DFS算法,找到连通区域:
```python
def dfs(i, j, visited, region):
visited[i][j] = True
region.append((i, j))
for di, dj in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
ni, nj = i+di, j+dj
if ni >= 0 and ni < visited.shape[0] and nj >= 0 and nj < visited.shape[1]:
if not visited[ni][nj] and gray[ni][nj] == gray[i][j]:
dfs(ni, nj, visited, region)
```
最后,遍历所有像素并进行DFS搜索,找到所有的连通区域:
```python
visited = np.zeros(gray.shape, dtype=bool)
regions = []
for i in range(visited.shape[0]):
for j in range(visited.shape[1]):
if not visited[i][j]:
region = []
dfs(i, j, visited, region)
if len(region) > 0:
regions.append(region)
```
完整的代码如下:
```python
import numpy as np
import cv2
def dfs(i, j, visited, region):
visited[i][j] = True
region.append((i, j))
for di, dj in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
ni, nj = i+di, j+dj
if ni >= 0 and ni < visited.shape[0] and nj >= 0 and nj < visited.shape[1]:
if not visited[ni][nj] and gray[ni][nj] == gray[i][j]:
dfs(ni, nj, visited, region)
img = cv2.imread('image.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
visited = np.zeros(gray.shape, dtype=bool)
regions = []
for i in range(visited.shape[0]):
for j in range(visited.shape[1]):
if not visited[i][j]:
region = []
dfs(i, j, visited, region)
if len(region) > 0:
regions.append(region)
print('Number of regions:', len(regions))
```
注意:这段代码只是一个简单的实现,如果要使用在对精度要求高的实际应用中,可能需要进行更多的优化和调整。
相关推荐
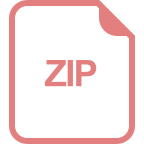
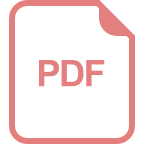














