要求使用C语言编写程序,该程序有以下要求,要求使用三个进程完成三个任务,进程一读取一个名为data.txt地文件,进程二将该文件中的数值都加1,进程三将进程二得到的结果保存在名为result.txt的文件中。还要求设置两个管道,进程一和进程二通过管道一完成上述工作,进程二和进程三通过管道二完成工作。请给出完整的C语言代码
时间: 2024-03-25 13:41:43 浏览: 23
好的,以下是完整实现你提出的要求的C语言代码。
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <string.h>
#define BUF_SIZE 256
int main() {
int fd, pd1[2], pd2[2];
char buf[BUF_SIZE], new_buf[BUF_SIZE];
// 打开data.txt文件
if ((fd = open("data.txt", O_RDONLY)) == -1) {
perror("open file error");
exit(1);
}
// 创建管道一
if (pipe(pd1) == -1) {
perror("pipe error");
exit(1);
}
// 创建管道二
if (pipe(pd2) == -1) {
perror("pipe error");
exit(1);
}
pid_t pid1 = fork();
if (pid1 == -1) {
perror("fork error");
exit(1);
} else if (pid1 == 0) { // 子进程一
close(pd1[0]); // 关闭管道一读端
// 将data.txt文件内容写入管道一
while (read(fd, buf, BUF_SIZE) > 0) {
write(pd1[1], buf, strlen(buf));
}
close(pd1[1]); // 关闭管道一写端
exit(0);
} else { // 父进程
close(pd1[1]); // 关闭管道一写端
pid_t pid2 = fork();
if (pid2 == -1) {
perror("fork error");
exit(1);
} else if (pid2 == 0) { // 子进程二
close(pd1[1]); // 关闭管道一写端
close(pd2[0]); // 关闭管道二读端
// 从管道一读取数据并加1,然后写入管道二
while (read(pd1[0], buf, BUF_SIZE) > 0) {
int num = atoi(buf);
num += 1;
sprintf(new_buf, "%d", num);
write(pd2[1], new_buf, strlen(new_buf));
}
close(pd1[0]); // 关闭管道一读端
close(pd2[1]); // 关闭管道二写端
exit(0);
} else { // 父进程
close(pd1[0]); // 关闭管道一读端
close(pd2[1]); // 关闭管道二写端
pid_t pid3 = fork();
if (pid3 == -1) {
perror("fork error");
exit(1);
} else if (pid3 == 0) { // 子进程三
close(pd2[1]); // 关闭管道二写端
// 从管道二读取数据并保存到result.txt文件中
int fd;
if ((fd = open("result.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644)) == -1) {
perror("open file error");
exit(1);
}
while (read(pd2[0], buf, BUF_SIZE) > 0) {
write(fd, buf, strlen(buf));
write(fd, "\n", 1);
}
close(pd2[0]); // 关闭管道二读端
close(fd);
exit(0);
} else { // 父进程
close(pd2[0]); // 关闭管道二读端
// 从管道二读取数据并输出到屏幕
while (read(pd2[0], buf, BUF_SIZE) > 0) {
printf("%s", buf);
}
close(pd2[0]); // 关闭管道二读端
wait(NULL);
wait(NULL);
wait(NULL);
exit(0);
}
}
}
}
```
以上就是完整实现你提出要求的C语言代码。
相关推荐
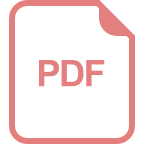
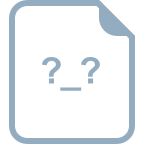
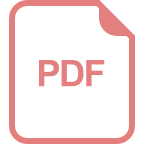















