用python读写xml文件
时间: 2023-03-27 22:03:11 浏览: 178
可以使用Python内置的xml库来读写xml文件,具体操作可以参考以下代码:
# 导入xml库
import xml.etree.ElementTree as ET
# 读取xml文件
tree = ET.parse('example.xml')
root = tree.getroot()
# 遍历xml文件
for child in root:
print(child.tag, child.attrib)
# 修改xml文件
for country in root.findall('country'):
rank = int(country.find('rank').text)
if rank > 50:
root.remove(country)
# 写入xml文件
tree.write('output.xml')
相关问题
python 读取xml文件
Python有许多用于读取XML文件的模块,其中最常用的是`ElementTree`模块。
以下是一个简单的例子,演示如何使用`ElementTree`模块读取XML文件:
```python
import xml.etree.ElementTree as ET
# 解析XML文件
tree = ET.parse('example.xml')
# 获取根元素
root = tree.getroot()
# 遍历XML文件并打印元素和其文本内容
for elem in root.iter():
print(elem.tag, elem.text)
```
在上面的例子中,我们首先使用`ET.parse()`方法解析XML文件。然后,我们使用`tree.getroot()`方法获取根元素,并使用`root.iter()`方法遍历XML文件中的所有元素。最后,我们打印每个元素的标签和文本内容。
注意:在使用`ElementTree`模块读取XML文件时,如果XML文件中包含命名空间,则需要对元素标签进行命名空间处理。
python 读取xml文件内容
可以使用Python内置的minidom模块来读取和解析XML文件内容。具体可以参考以下代码示例:
```python
from xml.dom import minidom
# 打开xml文件,获取dom对象
dom = minidom.parse('example.xml')
# 获取根节点
root_node = dom.documentElement
# 获取节点列表
books = root_node.getElementsByTagName('book')
# 遍历节点列表,获取节点属性和文本内容
for book in books:
print(f'Book ID: {book.getAttribute("id")}')
print(f'Book Title: {book.getElementsByTagName("title")[0].childNodes[0].data}')
print(f'Book Author: {book.getElementsByTagName("author")[0].childNodes[0].data}')
```
PS:这里的example.xml是一个样例XML文件,可以根据实际需要替换成自己的XML文件名。
阅读全文
相关推荐
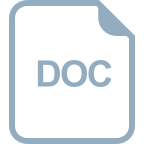
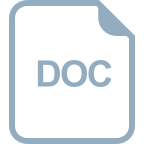
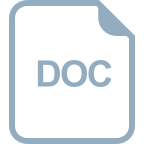
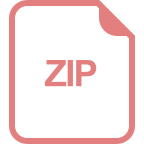
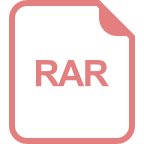
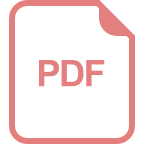
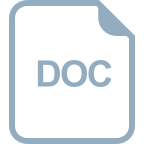
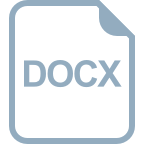





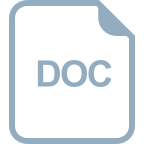
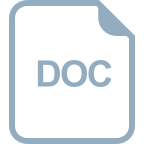
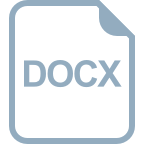