用c语言编写程序:要求两个线程共用一个函数同时读写一个串口,并不会产生死锁. 串口读写函数是先给串口发送一字符串,while循环一直等待串口接收数据,接收到数据退出循环
时间: 2024-01-24 10:17:53 浏览: 87
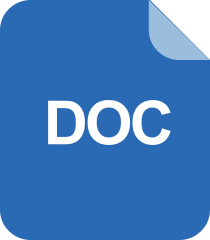
关于串口读写数据的文章
下面是一个示例程序,其中包含两个线程共用一个函数同时读写一个串口,不会产生死锁。需要注意的是,在使用串口的时候需要进行加锁和解锁操作,以确保数据传输的安全性。
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define PORT "/dev/ttyUSB0" // 串口设备文件路径
#define BAUDRATE B9600 // 波特率
pthread_mutex_t mutex; // 互斥锁,保证读写串口的安全性
void* serial_thread(void* args) // 串口读写线程函数
{
int fd;
char buf[256];
struct termios options;
fd = open(PORT, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0) {
perror("open");
return NULL;
}
fcntl(fd, F_SETFL, 0);
tcgetattr(fd, &options);
cfsetispeed(&options, BAUDRATE);
cfsetospeed(&options, BAUDRATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_cflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_oflag &= ~OPOST;
tcsetattr(fd, TCSANOW, &options);
while (1) {
pthread_mutex_lock(&mutex); // 加锁
write(fd, "hello", 5);
while (1) {
int len = read(fd, buf, 256);
if (len > 0) {
buf[len] = '\0';
printf("received: %s\n", buf);
break;
}
}
pthread_mutex_unlock(&mutex); // 解锁
}
close(fd);
return NULL;
}
int main()
{
pthread_t thread1, thread2;
pthread_mutex_init(&mutex, NULL);
pthread_create(&thread1, NULL, serial_thread, NULL);
pthread_create(&thread2, NULL, serial_thread, NULL);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
pthread_mutex_destroy(&mutex);
return 0;
}
```
在上述程序中,我们使用了互斥锁来保证串口的读写安全性。每个线程都会先获取锁,执行串口读写操作后再释放锁。这样能够避免多个线程同时访问串口造成的冲突问题。
阅读全文
相关推荐
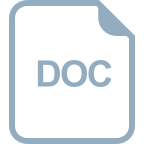
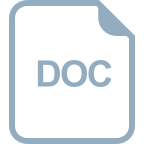
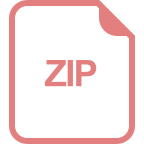
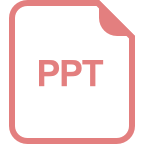
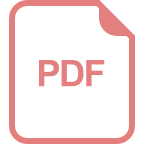
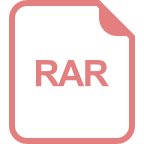
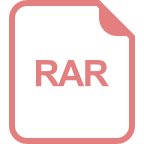
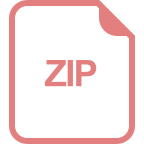
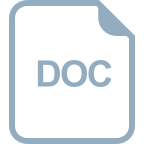
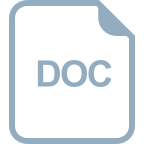
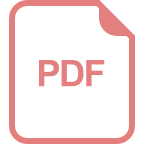
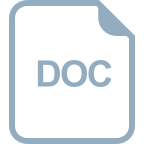
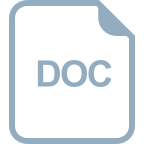
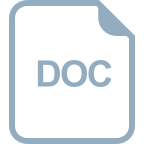
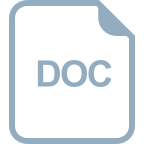
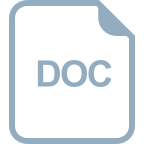

