java中对象深拷贝代码
时间: 2023-07-19 15:44:04 浏览: 93
下面是一个示例代码,用于实现 Java 中的对象深拷贝:
```
import java.io.*;
public class MyClass implements Cloneable, Serializable {
private static final long serialVersionUID = 1L;
private int myInt;
private String myString;
private MyObject myObject;
public MyClass(int myInt, String myString, MyObject myObject) {
this.myInt = myInt;
this.myString = myString;
this.myObject = myObject;
}
public Object clone() throws CloneNotSupportedException {
MyClass newObj = (MyClass) super.clone();
newObj.myObject = (MyObject) myObject.clone();
return newObj;
}
public MyClass deepCopy() throws IOException, ClassNotFoundException {
ByteArrayOutputStream bos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(bos);
oos.writeObject(this);
ByteArrayInputStream bis = new ByteArrayInputStream(bos.toByteArray());
ObjectInputStream ois = new ObjectInputStream(bis);
return (MyClass) ois.readObject();
}
}
class MyObject implements Cloneable, Serializable {
private static final long serialVersionUID = 1L;
private int myInt;
private String myString;
public MyObject(int myInt, String myString) {
this.myInt = myInt;
this.myString = myString;
}
public Object clone() throws CloneNotSupportedException {
return super.clone();
}
}
```
以上代码中,MyClass 类和 MyObject 类都实现了 Cloneable 接口,并重写了 clone() 方法。在 MyClass 类的 clone() 方法中,我们通过调用 super.clone() 方法首先创建了一个新的 MyClass 对象,然后将 myObject 属性也进行了深拷贝。在 MyClass 类的 deepCopy() 方法中,我们使用了序列化和反序列化的方式,将对象序列化为字节流,再将字节流反序列化为对象,以此实现了对象的深拷贝。
阅读全文
相关推荐
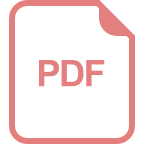
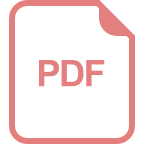
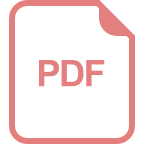















