java完成计算器的加、减、乘、除、开方、求反等功能,具体功能需求可以参考window自带计算器。完成对计算器界面开发的代码,完成计算器逻辑功能的开发
时间: 2023-12-12 12:02:50 浏览: 38
以下是一个简单的 Java 计算器实现,其中包含加、减、乘、除、开方、求反等功能:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator implements ActionListener {
private JFrame frame;
private JTextField textField;
private JButton addButton, subtractButton, multiplyButton, divideButton;
private JButton sqrtButton, negateButton, equalsButton, clearButton;
private double operand1, operand2;
private String operator;
public Calculator() {
frame = new JFrame("Calculator");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create the text field
textField = new JTextField(10);
textField.setEditable(false);
frame.getContentPane().add(textField, BorderLayout.NORTH);
// Create the buttons
addButton = new JButton("+");
subtractButton = new JButton("-");
multiplyButton = new JButton("*");
divideButton = new JButton("/");
sqrtButton = new JButton("sqrt");
negateButton = new JButton("+/-");
equalsButton = new JButton("=");
clearButton = new JButton("C");
// Add the buttons to the frame
JPanel buttonPanel = new JPanel();
buttonPanel.setLayout(new GridLayout(4, 4));
buttonPanel.add(addButton);
buttonPanel.add(subtractButton);
buttonPanel.add(multiplyButton);
buttonPanel.add(divideButton);
buttonPanel.add(sqrtButton);
buttonPanel.add(negateButton);
buttonPanel.add(equalsButton);
buttonPanel.add(clearButton);
for (int i = 1; i <= 9; i++) {
JButton button = new JButton(String.valueOf(i));
button.addActionListener(this);
buttonPanel.add(button);
}
JButton button = new JButton("0");
button.addActionListener(this);
buttonPanel.add(button);
frame.getContentPane().add(buttonPanel, BorderLayout.CENTER);
// Add action listeners to the buttons
addButton.addActionListener(this);
subtractButton.addActionListener(this);
multiplyButton.addActionListener(this);
divideButton.addActionListener(this);
sqrtButton.addActionListener(this);
negateButton.addActionListener(this);
equalsButton.addActionListener(this);
clearButton.addActionListener(this);
// Set the size and show the frame
frame.setSize(300, 300);
frame.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
String action = e.getActionCommand();
if (action.equals("C")) {
textField.setText("");
operand1 = 0;
operand2 = 0;
operator = "";
} else if (action.equals("+")) {
operand1 = Double.parseDouble(textField.getText());
operator = "+";
textField.setText("");
} else if (action.equals("-")) {
operand1 = Double.parseDouble(textField.getText());
operator = "-";
textField.setText("");
} else if (action.equals("*")) {
operand1 = Double.parseDouble(textField.getText());
operator = "*";
textField.setText("");
} else if (action.equals("/")) {
operand1 = Double.parseDouble(textField.getText());
operator = "/";
textField.setText("");
} else if (action.equals("sqrt")) {
operand1 = Double.parseDouble(textField.getText());
double result = Math.sqrt(operand1);
textField.setText(String.valueOf(result));
} else if (action.equals("+/-")) {
double value = Double.parseDouble(textField.getText());
value = -value;
textField.setText(String.valueOf(value));
} else if (action.equals("=")) {
operand2 = Double.parseDouble(textField.getText());
if (operator.equals("+")) {
double result = operand1 + operand2;
textField.setText(String.valueOf(result));
} else if (operator.equals("-")) {
double result = operand1 - operand2;
textField.setText(String.valueOf(result));
} else if (operator.equals("*")) {
double result = operand1 * operand2;
textField.setText(String.valueOf(result));
} else if (operator.equals("/")) {
double result = operand1 / operand2;
textField.setText(String.valueOf(result));
}
operand1 = 0;
operand2 = 0;
operator = "";
} else {
String value = textField.getText();
value += action;
textField.setText(value);
}
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
}
}
```
这个计算器界面比较简单,只包含了数字按钮、加、减、乘、除、开方、求反、等于和清除按钮。当用户点击数字和操作符按钮时,程序会根据当前的操作符和操作数计算出结果并更新文本框的显示内容。
相关推荐
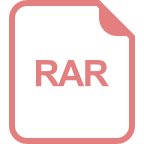














