//初始化时,如果是自定义的配置项,保存在this.customList里 const customIndex = this.customList.findIndex((el) => el.tips === item.name) if (customIndex !== -1) { const customItem = deepCopy(this.customList[customIndex]) this.$set(this.customList, customIndex, { ...customItem, ...item }) return result } 优化简便下代码
时间: 2024-03-16 12:42:10 浏览: 18
可以考虑使用对象解构和三元表达式来简化代码:
```
const customIndex = this.customList.findIndex(el => el.tips === item.name);
const customItem = customIndex !== -1 ? { ...deepCopy(this.customList[customIndex]), ...item } : item;
this.customList.splice(customIndex, 1, customItem);
return result;
```
这样就不需要再使用 `$set` 方法了,直接使用 `splice` 方法对数组进行修改即可。另外,使用对象解构可以将原来的两次赋值合并为一行代码。
相关问题
在vue中this.list=this.list1,并读取list数据时,怎么先对list数据排序
你可以使用JavaScript的`sort()`方法对数组进行排序,然后再将排序后的数组赋值给`this.list`。`sort()`方法接收一个函数作为参数,用于定义排序规则。例如,如果你想按照数组元素的数字大小进行升序排序,可以这样写:
```
this.list.sort((a, b) => a - b); // 升序排序
```
如果你想按照其他属性进行排序,可以根据需要自定义排序函数。例如,如果你的数组元素是对象,你可以按照对象的某个属性进行排序,例如:
```
this.list.sort((a, b) => a.name.localeCompare(b.name)); // 按照name属性进行排序
```
在这个例子中,我们使用`localeCompare()`方法对字符串进行排序,你可以根据实际情况选择合适的排序方法。
最后,将排序后的数组赋值给`this.list`即可。完整的代码可能如下所示:
```
methods: {
updateList() {
if (this.selectedItem === 'option3' && this.selectedYear === 'option1' && this.selectedTime === 'option1' && this.selectedIndex === 'option1') {
const sortedList = this.list1.slice().sort(); // 复制并排序
this.list = sortedList; // 赋值给响应式属性
}
}
}
```
const index = this.cart.findIndex((cartItem) => cartItem.name === item.name);改进这段代码
这段代码可以改进为使用 `Array.prototype.findIndex()` 的箭头函数简写形式,如下所示:
```
const index = this.cart.findIndex(cartItem => cartItem.name === item.name);
```
这样可以让代码更加简洁易读。另外,如果你使用了ES6的解构赋值,也可以将代码改写为:
```
const { name } = item;
const index = this.cart.findIndex(({ name: cartItemName }) => cartItemName === name);
```
这样可以更清晰地表达代码的意图,同时避免了多次访问 `item.name` 的问题。
相关推荐
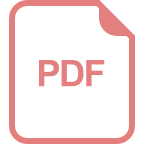
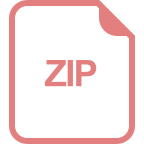
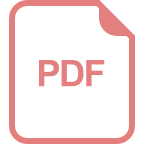












