c++文件管理系统
时间: 2023-07-24 16:11:48 浏览: 48
文件管理系统通常需要实现以下功能:
1. 文件的创建、复制、删除等基本操作
2. 文件夹的创建、复制、删除等基本操作
3. 文件和文件夹的重命名操作
4. 文件和文件夹的移动操作
5. 文件和文件夹的搜索操作
6. 文件和文件夹的信息查看操作
以下是一个简单的 C++ 文件管理系统的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
using namespace std;
void createFile(string filePath) {
ofstream file;
file.open(filePath);
file.close();
cout << "File created successfully!" << endl;
}
void copyFile(string sourcePath, string destPath) {
ifstream source(sourcePath, ios::binary);
ofstream dest(destPath, ios::binary);
dest << source.rdbuf();
cout << "File copied successfully!" << endl;
}
void deleteFile(string filePath) {
if (remove(filePath.c_str()) != 0) {
cout << "Error deleting file!" << endl;
} else {
cout << "File deleted successfully!" << endl;
}
}
void createDirectory(string dirPath) {
if (mkdir(dirPath.c_str(), 0777) != 0) {
cout << "Error creating directory!" << endl;
} else {
cout << "Directory created successfully!" << endl;
}
}
void copyDirectory(string sourcePath, string destPath) {
DIR* dir = opendir(sourcePath.c_str());
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
string fileName = entry->d_name;
if (fileName != "." && fileName != "..") {
string sourceFilePath = sourcePath + "/" + fileName;
string destFilePath = destPath + "/" + fileName;
struct stat s;
if (stat(sourceFilePath.c_str(), &s) == 0) {
if (s.st_mode & S_IFDIR) {
createDirectory(destFilePath);
copyDirectory(sourceFilePath, destFilePath);
} else {
copyFile(sourceFilePath, destFilePath);
}
}
}
}
closedir(dir);
cout << "Directory copied successfully!" << endl;
}
void deleteDirectory(string dirPath) {
DIR* dir = opendir(dirPath.c_str());
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
string fileName = entry->d_name;
if (fileName != "." && fileName != "..") {
string filePath = dirPath + "/" + fileName;
struct stat s;
if (stat(filePath.c_str(), &s) == 0) {
if (s.st_mode & S_IFDIR) {
deleteDirectory(filePath);
} else {
deleteFile(filePath);
}
}
}
}
closedir(dir);
if (rmdir(dirPath.c_str()) != 0) {
cout << "Error deleting directory!" << endl;
} else {
cout << "Directory deleted successfully!" << endl;
}
}
void renameFile(string oldFilePath, string newFilePath) {
if (rename(oldFilePath.c_str(), newFilePath.c_str()) != 0) {
cout << "Error renaming file!" << endl;
} else {
cout << "File renamed successfully!" << endl;
}
}
void renameDirectory(string oldDirPath, string newDirPath) {
if (rename(oldDirPath.c_str(), newDirPath.c_str()) != 0) {
cout << "Error renaming directory!" << endl;
} else {
cout << "Directory renamed successfully!" << endl;
}
}
void moveFile(string oldFilePath, string newFilePath) {
if (rename(oldFilePath.c_str(), newFilePath.c_str()) != 0) {
cout << "Error moving file!" << endl;
} else {
cout << "File moved successfully!" << endl;
}
}
void moveDirectory(string oldDirPath, string newDirPath) {
if (rename(oldDirPath.c_str(), newDirPath.c_str()) != 0) {
cout << "Error moving directory!" << endl;
} else {
cout << "Directory moved successfully!" << endl;
}
}
void searchFiles(string dirPath, string fileName) {
DIR* dir = opendir(dirPath.c_str());
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) {
string name = entry->d_name;
if (name.find(fileName) != string::npos) {
cout << dirPath << "/" << name << endl;
}
if (entry->d_type == DT_DIR && name != "." && name != "..") {
string filePath = dirPath + "/" + name;
searchFiles(filePath, fileName);
}
}
closedir(dir);
}
void viewFileInfo(string filePath) {
struct stat s;
if (stat(filePath.c_str(), &s) == 0) {
cout << "File size: " << s.st_size << " bytes" << endl;
cout << "Last modified time: " << ctime(&s.st_mtime);
} else {
cout << "Error getting file info!" << endl;
}
}
void viewDirInfo(string dirPath) {
DIR* dir = opendir(dirPath.c_str());
struct dirent* entry;
int fileCount = 0;
int dirCount = 0;
while ((entry = readdir(dir)) != NULL) {
if (entry->d_type == DT_DIR) {
dirCount++;
} else {
fileCount++;
}
}
closedir(dir);
cout << "File count: " << fileCount << endl;
cout << "Directory count: " << dirCount << endl;
}
int main() {
int choice;
string filePath, dirPath, oldFilePath, newFilePath, fileName;
while (true) {
cout << "1. Create file" << endl;
cout << "2. Copy file" << endl;
cout << "3. Delete file" << endl;
cout << "4. Create directory" << endl;
cout << "5. Copy directory" << endl;
cout << "6. Delete directory" << endl;
cout << "7. Rename file" << endl;
cout << "8. Rename directory" << endl;
cout << "9. Move file" << endl;
cout << "10. Move directory" << endl;
cout << "11. Search files" << endl;
cout << "12. View file info" << endl;
cout << "13. View directory info" << endl;
cout << "14. Exit" << endl;
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "Enter file path: ";
cin >> filePath;
createFile(filePath);
break;
case 2:
cout << "Enter source file path: ";
cin >> filePath;
cout << "Enter destination file path: ";
cin >> dirPath;
copyFile(filePath, dirPath);
break;
case 3:
cout << "Enter file path: ";
cin >> filePath;
deleteFile(filePath);
break;
case 4:
cout << "Enter directory path: ";
cin >> dirPath;
createDirectory(dirPath);
break;
case 5:
cout << "Enter source directory path: ";
cin >> dirPath;
cout << "Enter destination directory path: ";
cin >> filePath;
copyDirectory(dirPath, filePath);
break;
case 6:
cout << "Enter directory path: ";
cin >> dirPath;
deleteDirectory(dirPath);
break;
case 7:
cout << "Enter old file path: ";
cin >> oldFilePath;
cout << "Enter new file path: ";
cin >> newFilePath;
renameFile(oldFilePath, newFilePath);
break;
case 8:
cout << "Enter old directory path: ";
cin >> oldFilePath;
cout << "Enter new directory path: ";
cin >> newFilePath;
renameDirectory(oldFilePath, newFilePath);
break;
case 9:
cout << "Enter old file path: ";
cin >> oldFilePath;
cout << "Enter new file path: ";
cin >> newFilePath;
moveFile(oldFilePath, newFilePath);
break;
case 10:
cout << "Enter old directory path: ";
cin >> oldFilePath;
cout << "Enter new directory path: ";
cin >> newFilePath;
moveDirectory(oldFilePath, newFilePath);
break;
case 11:
cout << "Enter directory path: ";
cin >> dirPath;
cout << "Enter file name: ";
cin >> fileName;
searchFiles(dirPath, fileName);
break;
case 12:
cout << "Enter file path: ";
cin >> filePath;
viewFileInfo(filePath);
break;
case 13:
cout << "Enter directory path: ";
cin >> dirPath;
viewDirInfo(dirPath);
break;
case 14:
exit(0);
default:
cout << "Invalid choice!" << endl;
break;
}
}
return 0;
}
```
注意:这只是一个简单的示例代码,可能还需要更多的错误处理和安全性检查。
相关推荐
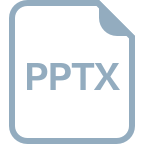













