c语言 实现soundex算法
时间: 2024-01-09 20:03:40 浏览: 151
Soundex算法是一种用于字符串匹配的算法,它可以将一个单词转换成一个固定长度的编码(通常为4个字符),以便于比较单词的相似性。以下是用C语言实现Soundex算法的代码示例:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
void soundex(char *s, char *soundex_code) {
const char *soundex_table = "01230120022455012623010202";
int i, j, prev_code, curr_code;
/* Convert the first letter to uppercase */
soundex_code[0] = toupper(s[0]);
/* Convert the remaining letters to their corresponding Soundex codes */
prev_code = soundex_table[s[0] - 'A'];
j = 1;
for (i = 1; i < strlen(s); i++) {
curr_code = soundex_table[s[i] - 'A'];
if (curr_code != '0' && curr_code != prev_code) {
soundex_code[j++] = curr_code;
}
prev_code = curr_code;
}
/* Pad the Soundex code with zeros if it is less than 4 characters */
while (j < 4) {
soundex_code[j++] = '0';
}
/* Terminate the string */
soundex_code[j] = '\0';
}
int main() {
char s[100], soundex_code[5];
printf("Enter a word: ");
fgets(s, sizeof(s), stdin);
s[strcspn(s, "\n")] = '\0';
soundex(s, soundex_code);
printf("Soundex code: %s\n", soundex_code);
return 0;
}
```
在这个示例中,我们首先定义了一个Soundex表格(soundex_table),它将字母映射到它们的Soundex代码。然后,我们实现了一个名为“soundex”的函数,它接受一个输入字符串和一个输出字符串作为参数,并将输入字符串转换为其对应的Soundex代码,存储在输出字符串中。在函数中,我们首先将输入字符串的第一个字母转换为大写字母,并将其存储在输出字符串的第一个位置上。然后,我们将剩余的字母转换为它们对应的Soundex代码,并将其存储在输出字符串中。如果两个相邻的字母具有相同的Soundex代码,则只存储一个代码。最后,我们将输出字符串填充到4个字符,并在结尾处添加一个空字符。在主函数中,我们从用户输入中获取一个单词,并将其传递给“soundex”函数,以获取其对应的Soundex代码。最后,我们将Soundex代码打印到控制台上。
阅读全文
相关推荐
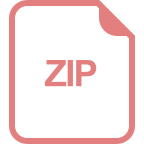
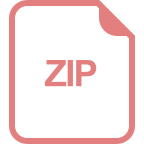
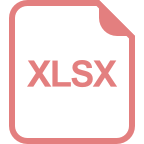
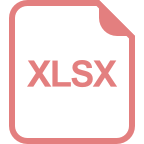
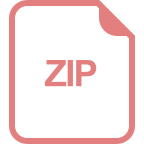
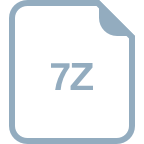
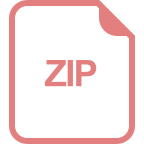
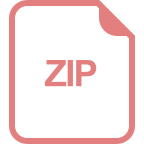
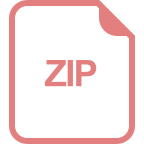
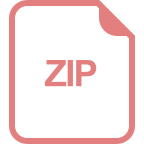