C++ Builder 6.0如何实现串口通信
时间: 2024-03-09 09:46:50 浏览: 148
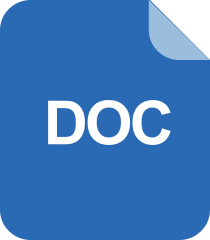
在Microsoft Visual C++ 6.0环境下通过对Active X控件的编程来实现串口的通信的一般方
C++ Builder 6.0可以通过使用Windows API来实现串口通信。以下是实现串口通信的基本步骤:
1. 打开串口:使用CreateFile函数打开串口设备文件,获取串口的句柄。
2. 配置串口参数:使用DCB结构体设置串口的波特率、数据位、停止位和校验位等参数。
3. 读写串口数据:使用ReadFile和WriteFile函数进行串口数据的读写操作。
4. 关闭串口:使用CloseHandle函数关闭串口句柄。
以下是代码示例:
```c++
#include <windows.h>
void serialComm()
{
HANDLE hSerial = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, 0, NULL,
OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (hSerial == INVALID_HANDLE_VALUE)
{
// 串口打开失败
return;
}
DCB dcbSerialParams = { 0 };
dcbSerialParams.DCBlength = sizeof(dcbSerialParams);
if (!GetCommState(hSerial, &dcbSerialParams))
{
// 获取串口参数失败
CloseHandle(hSerial);
return;
}
dcbSerialParams.BaudRate = CBR_9600;
dcbSerialParams.ByteSize = 8;
dcbSerialParams.StopBits = ONESTOPBIT;
dcbSerialParams.Parity = NOPARITY;
if (!SetCommState(hSerial, &dcbSerialParams))
{
// 设置串口参数失败
CloseHandle(hSerial);
return;
}
char buffer[1024] = { 0 };
DWORD bytesRead = 0;
DWORD bytesWritten = 0;
if (!ReadFile(hSerial, buffer, sizeof(buffer), &bytesRead, NULL))
{
// 读取串口数据失败
CloseHandle(hSerial);
return;
}
if (!WriteFile(hSerial, buffer, bytesRead, &bytesWritten, NULL))
{
// 写入串口数据失败
CloseHandle(hSerial);
return;
}
CloseHandle(hSerial);
}
```
阅读全文
相关推荐
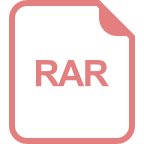
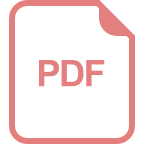
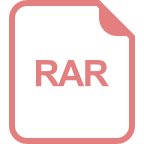
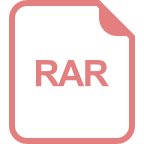
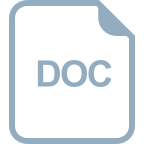
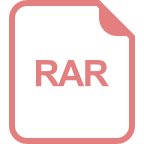
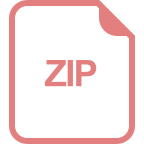
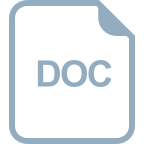
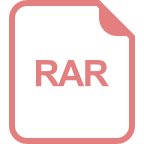
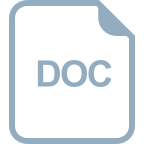
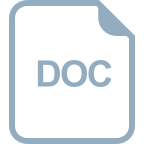
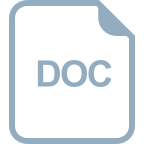
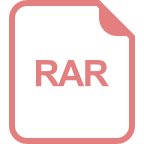