为什么用了请求转发,jsp页面的foreach的items还是显示无法解析
时间: 2024-05-31 21:07:26 浏览: 96
可能是因为数据没有被正确地传递到jsp页面中。请检查以下几个方面:
1. 确认请求转发的路径和jsp页面的路径是否正确。
2. 确认在请求转发之前,数据已经被正确地设置到request对象中。
3. 确认jsp页面中使用的变量名和request对象中设置的变量名是否一致。
4. 确认jsp页面中使用的标签库是否被正确地引入。
如果以上几个方面都没有问题,可以尝试在jsp页面中打印出request对象中的数据,以确认数据是否被正确地传递到jsp页面中。
相关问题
编写springMVC模拟数据请求,浏览器输入获得班级学生请求,服务器推送多个学生信息,并在页面中以复选框表格的形式显示;客户端勾选若干学生后提交数据,服务器获得学生信息后跳转到selected页面,以表格形式显示选中的学生列表代码
好的,以下是一个可能的代码实现:
班级类:
```java
public class Grade {
private int id;
private String name;
// 其他属性和getter/setter方法省略
}
```
学生类:
```java
public class Student {
private int id;
private String name;
private int age;
private String gender;
// 其他属性和getter/setter方法省略
}
```
Controller类:
```java
@Controller
@RequestMapping("/grade")
public class GradeController {
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public ModelAndView getStudents(@PathVariable int id) {
// 模拟从数据库中获取学生信息
List<Student> students = new ArrayList<Student>();
students.add(new Student(1, "张三", 18, "男"));
students.add(new Student(2, "李四", 19, "女"));
students.add(new Student(3, "王五", 20, "男"));
ModelAndView modelAndView = new ModelAndView("studentList");
modelAndView.addObject("students", students);
modelAndView.addObject("gradeId", id);
return modelAndView;
}
@RequestMapping(value = "/selected", method = RequestMethod.POST)
public ModelAndView selectedStudents(HttpServletRequest request) {
// 解析提交的学生信息
String[] selectedIds = request.getParameterValues("selectedIds");
List<Student> selectedStudents = new ArrayList<Student>();
for (String id : selectedIds) {
// 模拟从数据库中获取学生信息
selectedStudents.add(new Student(Integer.parseInt(id), "学生" + id, 18, "男"));
}
ModelAndView modelAndView = new ModelAndView("selectedStudents");
modelAndView.addObject("selectedStudents", selectedStudents);
return modelAndView;
}
}
```
studentList.jsp页面:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>班级学生列表</title>
</head>
<body>
<h1>班级学生列表</h1>
<form id="selectForm" action="${pageContext.request.contextPath}/grade/selected" method="post">
<input type="hidden" name="gradeId" value="${gradeId}" />
<table>
<thead>
<tr>
<th>选择</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<c:forEach var="student" items="${students}">
<tr>
<td><input type="checkbox" name="selectedIds" value="${student.id}" /></td>
<td>${student.name}</td>
<td>${student.age}</td>
<td>${student.gender}</td>
</tr>
</c:forEach>
</tbody>
</table>
<input type="submit" value="提交" />
</form>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script>
$(function() {
// 提交表单
$('#selectForm').submit(function() {
var selectCount = $('input[name="selectedIds"]:checked').length;
if (selectCount <= 0) {
alert('请选择要提交的学生!');
return false;
}
});
});
</script>
</body>
</html>
```
selectedStudents.jsp页面:
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>已选学生列表</title>
</head>
<body>
<h1>已选学生列表</h1>
<table>
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<c:forEach var="student" items="${selectedStudents}">
<tr>
<td>${student.name}</td>
<td>${student.age}</td>
<td>${student.gender}</td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
以上代码仅供参考,具体实现过程还需要根据具体的需求进行调整和实现。
阅读全文
相关推荐
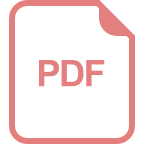
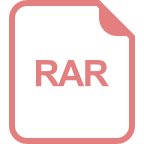
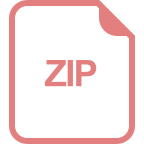
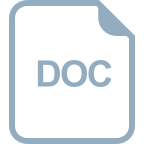
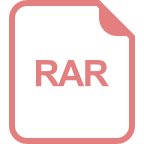
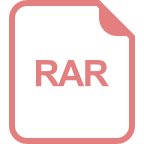
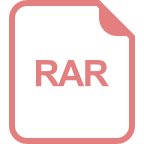
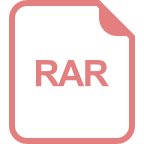
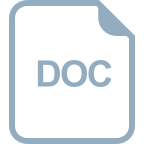
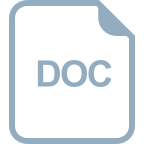
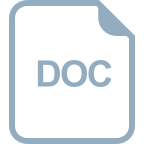
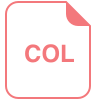
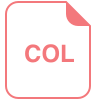
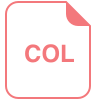
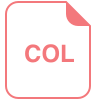
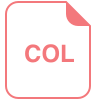
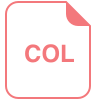
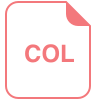
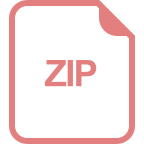