router.beforeEach((to, from, next) => { if (store.getters.getRouters == null) { // 获取菜单和路由 getMenusApi(params).then((res) => { if (res.data.length === 0) { window.location.href = process.env.VUE_APP_API_BASE_CONSOLE || '' } const { data } = res const menuNav = [] const childrenNav = [] // 后端数据, 根级树数组, 根级 PID listToTree(data, childrenNav, 0) menuNav.push(...childrenNav) const routers = generator(menuNav, null) routers.forEach((r) => { router.addRoute(r) }) router.addRoute(page404) store.commit('SET_ROUTERS', routers[0].children) // 请求带有 redirect 重定向时,登录自动重定向到该地址 const redirect = decodeURIComponent(from.query.redirect || to.path) if (to.path === redirect) { // set the replace: true so the navigation will not leave a history record next({ ...to, replace: true }) } else { // 跳转到目的路由 next({ path: redirect }) } }).catch((error) => { console.log(error,'error') if (error.data.code !== 200 || error.data.data.length === 0) { setTimeout(function () { window.location.href = process.env.VUE_APP_API_BASE_CONSOLE || '' }, 5) } }) // // 获取按钮权限 // getPermissionApi(params).then((res: any) => { // if (res.data.code !== 200) return ElMessage.error(res.data.message) // store.commit('SET_PERMISSION', res.data.data) // }) // // 获取数据权限 // getRolesApi(params).then((res: any) => { // if (res.data.code !== 200) return ElMessage.error(res.data.message) // store.commit('SET_ROLES', res.data.data) // }) // // 获取用户信息 // getUserInfoApi().then((res: any) => { // if (res.data.code !== 200) return ElMessage.error(res.data.message) // store.commit('SET_USERS', res.data.data) // }) } else { next() } })
时间: 2024-02-14 09:20:15 浏览: 30
这是一个 Vue 路由守卫的代码片段,用于在路由切换前进行一些操作。其中包含了以下主要逻辑:
1. 判断 store 中的路由信息是否为空,如果为空则向后端发起请求获取菜单和路由信息。
2. 通过 getMenusApi 函数向后端发起请求,获取菜单和路由信息。如果请求成功,则将获取到的菜单和路由信息转换为前端路由,并添加到 Vue Router 中。
3. 如果请求带有 redirect 重定向参数,则自动跳转到该地址。
4. 如果 store 中已经有路由信息,则直接跳转到目的路由。
5. 如果获取菜单和路由信息失败,则跳转到 404 页面或者登录页面。
注释中的代码是一些额外的逻辑,用于获取用户信息、按钮权限、数据权限等。
相关问题
import router from './router' import store from './store' import { Message } from 'element-ui' import NProgress from 'nprogress' import 'nprogress/nprogress.css' import { getToken } from '@/utils/auth' import { getInfo } from '@/api/login' NProgress.configure({ showSpinner: false }) const whiteList = ['/login', '/auth-redirect', '/bind', '/register','/homepage'] router.beforeEach((to, from, next) => { NProgress.start() if (getToken()) { to.meta.title && store.dispatch('settings/setTitle', to.meta.title) console.log("store.getters.roles====",store.getters.roles); console.log("getToken",getToken()) /* has token*/ if (to.path === '/login') { console.log("666666666") //判断身份非管理员跳转用户首页 if (store.getters.roles.length !== 0 && store.getters.roles.indexOf("admin") != -1 ) { console.log("7777777777777") next({ path: '/homepage' }) } else { console.log("88888888888888") next({ path: '/' }) } NProgress.done() } else { //其他路由 //角色未加载 if (store.getters.roles.length === 0) { // 判断当前用户是否已拉取完user_info信息 store.dispatch('GetInfo').then(() => { store.dispatch('GenerateRoutes').then(accessRoutes => { // 根据roles权限生成可访问的路由表 router.addRoutes(accessRoutes) // 动态添加可访问路由表 next({ ...to, replace: true }) // hack方法 确保addRoutes已完成 }) }).catch(err => { store.dispatch('LogOut').then(() => { Message.error(err) next({ path: '/' }) }) }) } else { next() } } } else { // 没有token if (whiteList.indexOf(to.path) !== -1) { // 在免登录白名单,直接进入 next() } else { next(`/homepage?redirect=${to.fullPath}`) // 否则全部重定向到登录页 NProgress.done() } } }) router.afterEach(() => { NProgress.done() }) 这段代码我想让 store.getters.roles.length 一定存在 怎么办
你可以在 Vuex 的 state 中设置一个默认的 roles 数组,在用户登录时就将后台返回的用户角色存储到这个数组中。这样在 beforeach 中判断 store.getters.roles.length 是否为 0 时,就不会出现不存在的情况了。同时也要注意在用户退出登录时清空这个 roles 数组。
router.beforeEach((to, from, next) => { if (!to.matched.length) { next('/404') } else { next() } })
这段代码是 Vue Router 中的全局前置守卫,作用是在路由跳转之前进行拦截和检查。如果 `to` 对象中的 `matched` 数组长度为 0,表示该路由不存在或者未定义,那么就调用 `next` 方法跳转至 404 页面。如果 `matched` 数组长度不为 0,说明该路由存在并已经匹配到了对应的组件,那么就调用 `next` 方法进行路由跳转。
相关推荐
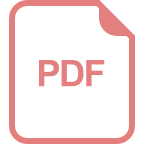
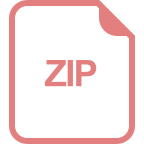
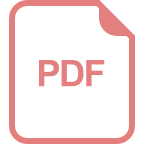













