可以后端用springboot,前端用angular,使用websocket和STOMP,实现一对一聊天和群聊的基础上,写一个监听消息是否已读的例子吗
时间: 2024-03-14 12:48:17 浏览: 161
好的,我们可以使用Spring Boot作为后端框架,Angular作为前端框架,使用WebSocket和STOMP协议来实现一对一聊天和群聊的功能,并编写一个监听消息是否已读的例子。
首先,我们需要在后端引入`spring-boot-starter-websocket`和`spring-boot-starter-data-jpa`依赖,并配置WebSocket和STOMP协议,代码如下:
```java
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void configureMessageBroker(MessageBrokerRegistry registry) {
registry.enableSimpleBroker("/topic");
registry.setApplicationDestinationPrefixes("/app");
}
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/chat").withSockJS();
}
}
```
其中,`configureMessageBroker()`方法配置了消息代理,`registerStompEndpoints()`方法注册了STOMP端点。
接着,我们可以编写一个控制器类,处理一对一聊天和群聊的请求,同时在消息发送时将消息保存到数据库中:
```java
@RestController
@RequestMapping("/chat")
public class ChatController {
@Autowired
private ChatMessageRepository chatMessageRepository;
@MessageMapping("/send")
@SendTo("/topic/messages")
public ChatMessage send(ChatMessage message) {
ChatMessage savedMessage = chatMessageRepository.save(message);
return savedMessage;
}
@MessageMapping("/group")
@SendTo("/topic/group")
public ChatMessage group(ChatMessage message) {
ChatMessage savedMessage = chatMessageRepository.save(message);
return savedMessage;
}
}
```
其中,`ChatMessage`是一个实体类,用于存储聊天消息到数据库中。
在前端方面,我们可以使用Angular和`@stomp/ng2-stompjs`来实现WebSocket连接和STOMP协议的使用。我们需要在模块中引入`@stomp/ng2-stompjs`模块,并在组件中注入`StompService`,然后使用`StompService`来创建一个连接,并通过`StompService.subscribe()`方法订阅消息,代码如下:
```typescript
import { Component, OnInit } from '@angular/core';
import { StompService } from '@stomp/ng2-stompjs';
@Component({
selector: 'app-chat',
templateUrl: './chat.component.html',
styleUrls: ['./chat.component.css']
})
export class ChatComponent implements OnInit {
private stompClient;
constructor(private stompService: StompService) { }
ngOnInit() {
this.stompClient = this.stompService.client;
this.stompClient.connect({}, () => {
this.stompClient.subscribe('/topic/messages', (message) => {
console.log(message.body);
});
});
}
sendMessage() {
const message = { content: 'Hello, world!' };
this.stompClient.send('/app/send', {}, JSON.stringify(message));
}
}
```
在以上代码中,我们使用了`ngOnInit()`方法来创建WebSocket连接,并使用`subscribe()`方法订阅服务器发送的消息。在发送消息时,我们使用`send()`方法发送一个JSON格式的消息到服务器中。
最后,我们需要编写一个监听消息是否已读的例子。我们可以在前端使用`StompService.subscribe()`方法订阅消息,然后在消息被读取时发送一个已读的标记到服务器中,代码如下:
```typescript
import { Component, OnInit } from '@angular/core';
import { StompService } from '@stomp/ng2-stompjs';
@Component({
selector: 'app-chat',
templateUrl: './chat.component.html',
styleUrls: ['./chat.component.css']
})
export class ChatComponent implements OnInit {
private stompClient;
constructor(private stompService: StompService) { }
ngOnInit() {
this.stompClient = this.stompService.client;
this.stompClient.connect({}, () => {
this.stompClient.subscribe('/topic/messages', (message) => {
console.log(message.body);
this.markAsRead(message.body.id); // 将消息标记为已读
});
});
}
markAsRead(id: number) {
const message = { id: id };
this.stompClient.send('/app/read', {}, JSON.stringify(message)); // 发送已读标记到服务器
}
}
```
在以上代码中,我们使用了`markAsRead()`方法将消息标记为已读,并使用`send()`方法将已读标记发送到服务器中。服务器收到已读标记后,可以将消息的状态设置为已读。
以上就是一个使用Spring Boot和Angular实现WebSocket和STOMP协议的一对一聊天和群聊的例子,并编写一个监听消息是否已读的例子的完整代码实现。
阅读全文
相关推荐
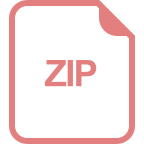
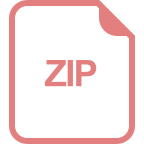

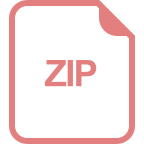
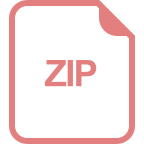
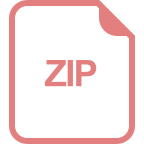
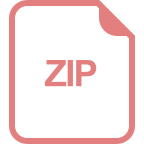
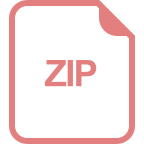
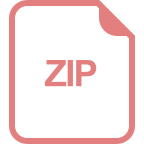
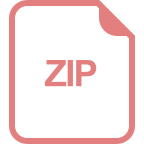
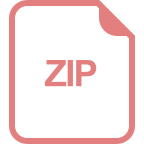
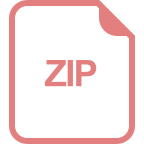
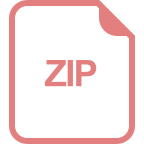
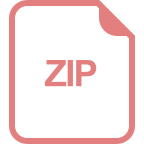
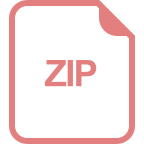
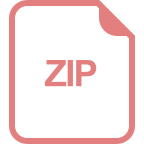
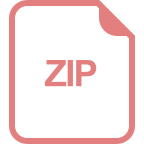
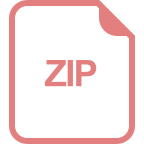