model = load_word2vec_model(w2v_path)之后如何获得词向量
时间: 2023-12-06 20:02:17 浏览: 77
在加载Word2Vec模型后,你可以使用`model.wv[word]`来获取某个词的词向量。以下是一个示例代码:
```python
from gensim.models import Word2Vec
# 加载预训练好的模型
model = Word2Vec.load("path/to/pretrained/model")
# 获取某个词的词向量
word = "apple"
vector = model.wv[word]
print(vector)
```
你可以将上面的代码中的`word`替换成你想要获取词向量的词,然后运行代码即可获得该词的词向量。注意,如果你想获取一个不存在于模型中的词的词向量,会抛出`KeyError`异常,因此需要在使用`model.wv[word]`之前确保该词在模型中存在。
相关问题
如何使用word2vec加载词向量
可以使用Python中的gensim库,通过以下几个步骤实现加载词向量:
1. 安装 gensim 库。在命令行中输入 pip install gensim。
2. 导入 gensim 库并加载预训练的词向量。例如:
```python
from gensim.models import KeyedVectors
# 加载预先训练好的词向量
w2v_model = KeyedVectors.load_word2vec_format('path/to/pretrained/word2vec/file', binary=True)
```
3. 使用已加载的词向量进行相应的自然语言处理任务。例如,可以获取某个单词的词向量表示:
```python
# 获取单词 "apple" 的词向量表示
word_vector = w2v_model.get_vector('apple')
```
或者,可以计算两个单词之间的相似度:
```python
# 计算单词 "apple" 和单词 "orange" 之间的相似度
similarity = w2v_model.similarity('apple', 'orange')
```
通过以上步骤,你就可以成功加载预训练的词向量,并使用它们进行相应的自然语言处理任务了。
Word2vec训练好的词向量如何加载到基于Keras的LSTM中
加载Word2vec训练好的词向量到基于Keras的LSTM中需要进行以下步骤:
1. 导入Word2vec模型,如:
```
from gensim.models import Word2Vec
w2v_model = Word2Vec.load('path/to/word2vec/model')
```
2. 获取Word2vec模型中的词向量矩阵,如:
```
embedding_matrix = w2v_model.wv.vectors
```
3. 构建Keras的Embedding层,将词向量矩阵作为权重传入,如:
```
from keras.layers import Embedding
embedding_layer = Embedding(input_dim=embedding_matrix.shape[0], output_dim=embedding_matrix.shape[1], weights=[embedding_matrix], trainable=False)
```
这里的 `input_dim` 参数为词汇表大小,`output_dim` 参数为词向量维度,`trainable` 参数表示词向量是否可被训练。
4. 在LSTM模型中使用Embedding层,如:
```
from keras.models import Sequential
from keras.layers import LSTM, Dense
model = Sequential()
model.add(embedding_layer)
model.add(LSTM(units=64, dropout=0.2, recurrent_dropout=0.2))
model.add(Dense(units=1, activation='sigmoid'))
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
```
这里的LSTM模型仅作为示例,具体模型结构和参数需根据实际情况进行调整。
通过以上步骤,即可将Word2vec训练好的词向量加载到基于Keras的LSTM模型中进行文本分类等任务。
阅读全文
相关推荐
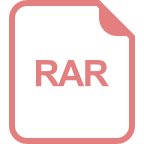
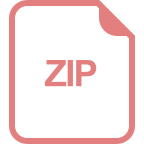








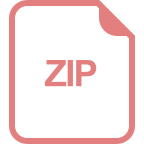
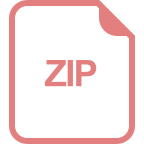
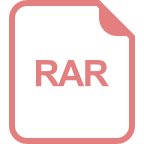
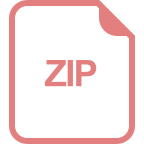
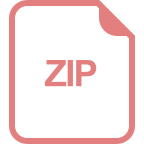
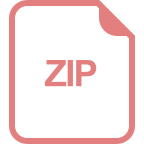