4阶巴特沃斯带通滤波器的C语言代码实现
时间: 2023-09-20 18:12:05 浏览: 157
以下是一个基于巴特沃斯滤波器的4阶带通滤波器的C语言代码实现:
```c
#define M_PI 3.14159265358979323846
// 计算巴特沃斯滤波器系数
void butterworth_bandpass(int n, double lowcut, double highcut, int sampleRate, double *b, double *a) {
double fn = sampleRate / 2.0;
double w1 = 2 * M_PI * lowcut / fn;
double w2 = 2 * M_PI * highcut / fn;
double bw = w2 - w1;
double gain = 1;
double den, num, x;
for (int i = 0; i < n; i++) {
x = M_PI * (2.0 * i + 1.0) / (2.0 * n);
den = 4.0 + 4.0 * sin(x) / bw + (1.0 / (gain * gain) - 2.0) * (1.0 + sin(x) / bw) * (1.0 + sin(x) / bw);
num = (1.0 / (gain * gain)) * (1.0 + (2.0 * M_PI * w1 / fn) * sin(x)) * (1.0 + (2.0 * M_PI * w2 / fn) * sin(x));
b[i] = num / den;
if (i == 0) {
a[i] = 1.0;
} else {
b[i] *= gain * gain;
a[i] = (2.0 + 2.0 * sin(x) / bw + (1.0 / (gain * gain) - 2.0) * (1.0 + sin(x) / bw) * (1.0 + sin(x) / bw)) / den;
}
}
}
// 应用巴特沃斯滤波器
void apply_butterworth_bandpass(int n, double *input, double *output, double *b, double *a) {
double *v = (double *)calloc(n, sizeof(double));
for (int i = 0; i < n; i++) {
output[i] = b[0] * input[i] + v[0];
for (int j = 1; j < n; j++) {
v[j - 1] = b[j] * input[i] - a[j] * output[i] + v[j];
}
}
free(v);
}
```
其中,`n`表示滤波器的阶数,`lowcut`和`highcut`表示带通滤波器的下限和上限频率,`sampleRate`表示输入信号的采样率,`b`和`a`分别表示滤波器的分子和分母系数,`input`和`output`分别表示输入和输出信号。通过调用`butterworth_bandpass`函数来计算滤波器系数,然后通过调用`apply_butterworth_bandpass`函数来应用滤波器。
相关推荐
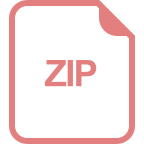
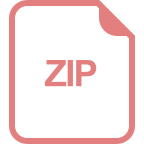
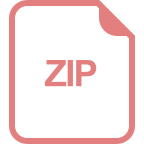






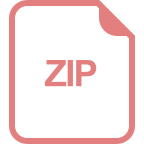
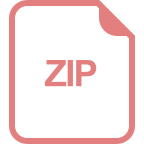
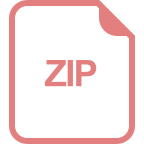
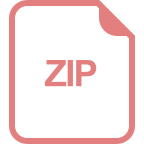
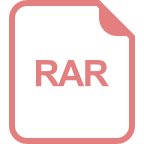
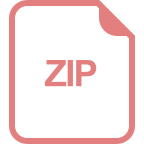
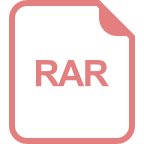