C#如何将txt文件读取进字符串后进行分割
时间: 2024-02-16 21:00:00 浏览: 49
使用C#可以使用StreamReader类来读取文本文件,然后将读取的文件内容存储在字符串变量中。对于分割字符串,可以使用Split方法。
以下是示例代码:
```csharp
string filePath = @"C:\example.txt"; // 文件路径
string fileContent = string.Empty; // 存储文件内容的字符串变量
// 读取文件内容
using (StreamReader sr = new StreamReader(filePath))
{
fileContent = sr.ReadToEnd();
}
// 对字符串进行分割
string[] splitContent = fileContent.Split(new char[] { ' ', '\r', '\n' }, StringSplitOptions.RemoveEmptyEntries);
// 输出分割结果
foreach (string s in splitContent)
{
Console.WriteLine(s);
}
```
在这个示例中,我们首先使用StreamReader类读取指定的文本文件,然后将读取的内容存储在字符串变量fileContent中。接着,我们使用Split方法将字符串分割为数组,使用空格、回车符和换行符作为分隔符。最后,我们遍历分割后的数组并输出结果。
相关问题
C#如何将导入的txt文件分割成数组
你可以使用C#中的`File`和`String`类来读取和处理文本文件。下面是一个简单的示例代码,展示如何将一个文本文件的每一行分割成一个字符串数组:
```csharp
using System;
using System.IO;
class Program
{
static void Main(string[] args)
{
// 读取文本文件的路径
string filePath = @"C:\example\file.txt";
// 读取文本文件的每一行,存储到字符串数组中
string[] lines = File.ReadAllLines(filePath);
// 分割每一行成一个字符串数组
foreach (string line in lines)
{
string[] words = line.Split(' '); // 使用空格分割每一行的字符串
// 输出每个字符串数组元素
foreach (string word in words)
{
Console.WriteLine(word);
}
}
}
}
```
在这个例子中,我们首先使用`File.ReadAllLines()`方法读取文本文件的每一行,并将它们存储到一个字符串数组中。接下来,我们对于每一行,使用`Split()`方法将它分割成一个字符串数组,其中分割符为一个空格。最后,我们遍历每个字符串数组元素,并输出到控制台。
用C#将已读取的txt文件并导入到已有的六列datagridview1控件中
可以按照以下步骤来实现:
1.读取txt文件内容并保存到一个字符串数组中。
```c#
string[] lines = File.ReadAllLines("文件路径");
```
2.将字符串数组中的内容逐行分割,并保存到一个二维数组中。
```c#
string[][] data = new string[lines.Length][];
for (int i = 0; i < lines.Length; i++)
{
data[i] = lines[i].Split(',');
}
```
3.将二维数组中的内容逐行添加到DataGridView控件中。
```c#
for (int i = 0; i < data.Length; i++)
{
dataGridView1.Rows.Add(data[i]);
}
```
完整代码示例:
```c#
string[] lines = File.ReadAllLines("文件路径");
string[][] data = new string[lines.Length][];
for (int i = 0; i < lines.Length; i++)
{
data[i] = lines[i].Split(',');
}
for (int i = 0; i < data.Length; i++)
{
dataGridView1.Rows.Add(data[i]);
}
```
相关推荐
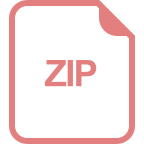
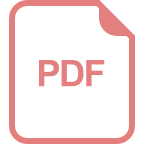
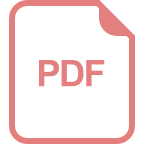
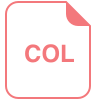
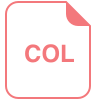










