在IDEA中用JAVA设计一个教材类,一个课程类,及一个为某门课程指定参考教材的类。一门课程可以有多本参考教材,再设计一个测试类。 设计一个简单的学生选课系统,并可以完成添加、删除、修改、查询相关信息 。 说明: 可以设计以下类: 描述课程基本信息类(课程编号,课程名,学分 ); 描述学生基本信息类(学号,姓名,专业方向 ); 记录选课结果的类(课程,选课的学生,课程最多人数,当前人数 ); 测试类(设定或输入最多人数,创建课程对象,创建选课学生对象,创建选课对象,显示选课情况 )
时间: 2024-03-03 12:53:55 浏览: 88
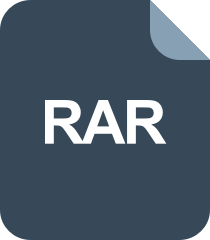
JAVA课程设计看看吧
好的,我可以帮您解答这个问题。下面是代码实现:
教材类:
```java
public class Textbook {
private String name; // 教材名称
private String author; // 作者
private double price; // 价格
public Textbook(String name, String author, double price) {
this.name = name;
this.author = author;
this.price = price;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public double getPrice() {
return price;
}
}
```
课程类:
```java
import java.util.ArrayList;
public class Course {
private String id; // 课程编号
private String name; // 课程名称
private int credit; // 学分
private ArrayList<Textbook> textbooks; // 参考教材
public Course(String id, String name, int credit) {
this.id = id;
this.name = name;
this.credit = credit;
textbooks = new ArrayList<>();
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public int getCredit() {
return credit;
}
public void addTextbook(Textbook textbook) {
textbooks.add(textbook);
}
public ArrayList<Textbook> getTextbooks() {
return textbooks;
}
}
```
为某门课程指定参考教材的类:
```java
public class CourseTextbook {
private Course course; // 课程
private Textbook textbook; // 参考教材
public CourseTextbook(Course course, Textbook textbook) {
this.course = course;
this.textbook = textbook;
course.addTextbook(textbook);
}
public Course getCourse() {
return course;
}
public Textbook getTextbook() {
return textbook;
}
}
```
学生基本信息类:
```java
public class Student {
private String id; // 学号
private String name; // 姓名
private String major; // 专业方向
public Student(String id, String name, String major) {
this.id = id;
this.name = name;
this.major = major;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public String getMajor() {
return major;
}
}
```
记录选课结果的类:
```java
import java.util.ArrayList;
public class CourseSelection {
private Course course; // 课程
private int maxNum; // 课程最多人数
private ArrayList<Student> students; // 选课的学生
public CourseSelection(Course course, int maxNum) {
this.course = course;
this.maxNum = maxNum;
students = new ArrayList<>();
}
public boolean addStudent(Student student) {
if (students.size() >= maxNum) {
return false;
}
students.add(student);
return true;
}
public boolean removeStudent(Student student) {
return students.remove(student);
}
public ArrayList<Student> getStudents() {
return students;
}
}
```
测试类:
```java
public class Test {
public static void main(String[] args) {
// 设定或输入最多人数
int maxNum = 50;
// 创建课程对象
Course math = new Course("001", "高等数学", 3);
Course physics = new Course("002", "大学物理", 4);
// 创建参考教材对象
Textbook mathTextbook1 = new Textbook("高等数学(第一册)", "李永乐", 50);
Textbook mathTextbook2 = new Textbook("高等数学(第二册)", "李永乐", 60);
Textbook physicsTextbook1 = new Textbook("大学物理(第一册)", "张伯礼", 80);
// 为课程指定参考教材
CourseTextbook mathTextbook = new CourseTextbook(math, mathTextbook1);
CourseTextbook mathTextbook2 = new CourseTextbook(math, mathTextbook2);
CourseTextbook physicsTextbook = new CourseTextbook(physics, physicsTextbook1);
// 创建选课学生对象
Student student1 = new Student("1001", "张三", "计算机科学");
Student student2 = new Student("1002", "李四", "计算机科学");
Student student3 = new Student("1003", "王五", "软件工程");
// 创建选课对象
CourseSelection mathSelection = new CourseSelection(math, maxNum);
CourseSelection physicsSelection = new CourseSelection(physics, maxNum);
// 添加选课学生
mathSelection.addStudent(student1);
mathSelection.addStudent(student2);
mathSelection.addStudent(student3);
physicsSelection.addStudent(student1);
physicsSelection.addStudent(student2);
// 显示选课情况
System.out.println(math.getName() + "选课情况:");
System.out.println("课程编号:" + math.getId());
System.out.println("学分:" + math.getCredit());
System.out.println("参考教材:");
ArrayList<Textbook> mathTextbooks = math.getTextbooks();
for (Textbook textbook : mathTextbooks) {
System.out.println(textbook.getName() + " " + textbook.getAuthor() + " " + textbook.getPrice());
}
System.out.println("选课人数:" + mathSelection.getStudents().size());
System.out.println("选课学生:");
ArrayList<Student> mathStudents = mathSelection.getStudents();
for (Student student : mathStudents) {
System.out.println(student.getId() + " " + student.getName() + " " + student.getMajor());
}
System.out.println();
System.out.println(physics.getName() + "选课情况:");
System.out.println("课程编号:" + physics.getId());
System.out.println("学分:" + physics.getCredit());
System.out.println("参考教材:");
ArrayList<Textbook> physicsTextbooks = physics.getTextbooks();
for (Textbook textbook : physicsTextbooks) {
System.out.println(textbook.getName() + " " + textbook.getAuthor() + " " + textbook.getPrice());
}
System.out.println("选课人数:" + physicsSelection.getStudents().size());
System.out.println("选课学生:");
ArrayList<Student> physicsStudents = physicsSelection.getStudents();
for (Student student : physicsStudents) {
System.out.println(student.getId() + " " + student.getName() + " " + student.getMajor());
}
}
}
```
通过上述代码实现,我们就可以简单地设计一个学生选课系统,并可以完成添加、删除、修改、查询相关信息。
阅读全文
相关推荐
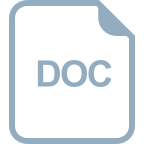
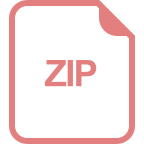
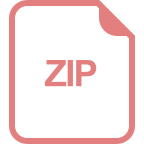
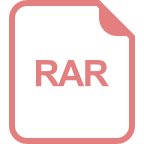
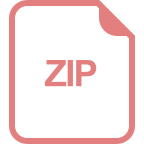
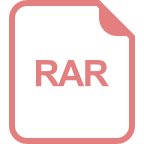
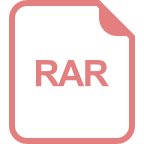
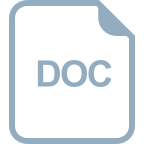
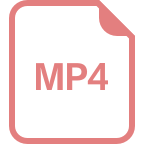
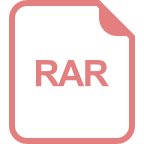
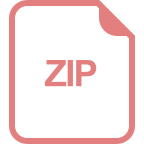
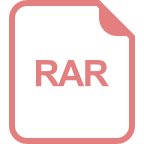
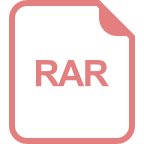
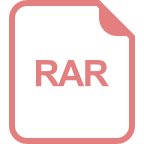
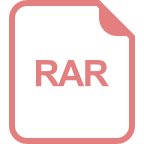
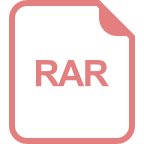