nuxt $router跳转传参
时间: 2023-09-11 19:03:59 浏览: 50
在 Nuxt.js 中,我们可以使用 `$router` 对象来进行路由跳转和传递参数。
假设我们要跳转到一个名为 `post` 的路由,并且需要传递一个名为 `id` 的参数,我们可以这样写:
```javascript
this.$router.push({ path: '/post', query: { id: postId }})
```
其中,`path` 表示路由路径,`query` 是一个对象,表示要传递的参数。在这个例子中,我们传递了一个名为 `id` 的参数,它的值是变量 `postId`。
在目标路由组件中,我们可以通过 `$route.query` 来获取传递的参数:
```javascript
export default {
mounted() {
const id = this.$route.query.id
// do something with id
}
}
```
注意,如果要在 `$route` 中获取动态路由参数,可以使用 `$route.params`。例如,如果我们的路由路径是 `/post/:id`,那么就可以这样获取参数:
```javascript
const id = this.$route.params.id
```
相关问题
nuxt-link跳转传参
在 Nuxt 中使用 `nuxt-link` 跳转传参可以通过以下两种方式实现:
1. 使用 `$route` 对象
在组件中使用 `$route` 对象获取当前路由信息,然后通过 `query` 或 `params` 对象来传递参数。
例如:
```html
<template>
<div>
<nuxt-link :to="{ name: 'my-page', query: { id: 123, name: 'test' } }">跳转到我的页面</nuxt-link>
</div>
</template>
```
在目标页面中可以通过 `$route.query` 获取传递的参数。
例如:
```js
export default {
mounted() {
console.log(this.$route.query.id) // 123
console.log(this.$route.query.name) // test
}
}
```
2. 使用动态路由
在路由配置中使用动态路由来传递参数。
例如:
```js
// 路由配置
{
path: '/my-page/:id',
name: 'my-page',
component: MyPage
}
```
在组件中使用 `params` 对象来传递参数。
例如:
```html
<template>
<div>
<nuxt-link :to="{ name: 'my-page', params: { id: 123 } }">跳转到我的页面</nuxt-link>
</div>
</template>
```
在目标页面中可以通过 `$route.params` 获取传递的参数。
例如:
```js
export default {
mounted() {
console.log(this.$route.params.id) // 123
}
}
```
nuxt3 router.push传参
在Nuxt.js 3中,可以通过`router.push`方法来传递参数。你可以使用两种方式来传递参数:使用`query`或者使用`params`。
使用`query`传递参数的方式如下:
```javascript
this.$router.push({ path: '/your-path', query: { paramName: paramValue } });
```
其中,`your-path`是你要跳转到的路径,`paramName`是参数的名称,`paramValue`是参数的值。
使用`params`传递参数的方式如下:
```javascript
this.$router.push({ name: 'your-route-name', params: { paramName: paramValue } });
```
其中,`your-route-name`是你要跳转到的路由的名称,`paramName`是参数的名称,`paramValue`是参数的值。
当你在目标页面中接收参数时,可以使用`$route.query.paramName`来接收`query`传递的参数,或者使用`$route.params.paramName`来接收`params`传递的参数。
请注意,以上示例中的`your-path`和`your-route-name`应该根据你的实际需求进行替换。
相关推荐
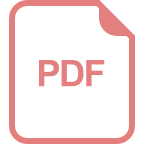
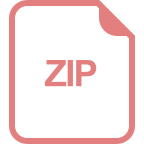
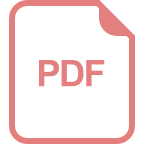












