winform 使用socket编写tcpip协议
时间: 2023-07-23 11:32:54 浏览: 136
要使用WinForm编写TCP/IP协议的程序,需要使用Socket类。下面是一个简单的示例代码,演示如何使用Socket类发送和接收数据。
```csharp
using System;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Windows.Forms;
namespace TcpIpDemo
{
public partial class Form1 : Form
{
private Socket clientSocket;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
}
private void btnConnect_Click(object sender, EventArgs e)
{
try
{
// 连接服务器
clientSocket.Connect(new IPEndPoint(IPAddress.Parse(txtServerIP.Text), int.Parse(txtServerPort.Text)));
txtLog.AppendText("连接成功!\n");
}
catch (Exception ex)
{
txtLog.AppendText("连接失败:" + ex.Message + "\n");
return;
}
// 启动接收数据的线程
ReceiveThreadStart();
}
private void btnSend_Click(object sender, EventArgs e)
{
try
{
// 发送数据
byte[] data = Encoding.UTF8.GetBytes(txtInput.Text);
clientSocket.Send(data);
}
catch (Exception ex)
{
txtLog.AppendText("发送失败:" + ex.Message + "\n");
return;
}
}
private void ReceiveThreadStart()
{
// 启动接收数据的线程
System.Threading.Thread receiveThread = new System.Threading.Thread(ReceiveThreadFunc);
receiveThread.IsBackground = true;
receiveThread.Start();
}
private void ReceiveThreadFunc()
{
byte[] buffer = new byte[1024];
while (true)
{
try
{
// 接收数据
int count = clientSocket.Receive(buffer);
if (count > 0)
{
string msg = Encoding.UTF8.GetString(buffer, 0, count);
this.Invoke((Action)(() =>
{
txtLog.AppendText(msg + "\n");
}));
}
else
{
break;
}
}
catch (Exception ex)
{
txtLog.AppendText("接收数据出错:" + ex.Message + "\n");
break;
}
}
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
try
{
// 关闭连接
clientSocket.Shutdown(SocketShutdown.Both);
clientSocket.Close();
}
catch (Exception ex)
{
txtLog.AppendText("关闭连接出错:" + ex.Message + "\n");
}
}
}
}
```
在上面的示例代码中,我们使用了一个Socket对象来代表客户端,使用Connect方法来连接服务器。发送数据时,我们使用了Send方法,接收数据时,我们使用了Receive方法。为了能够在接收数据时更新UI,我们使用了Invoke方法来执行UI线程上的操作。
当窗口关闭时,我们使用Shutdown方法来关闭连接,并使用Close方法来释放资源。
阅读全文
相关推荐
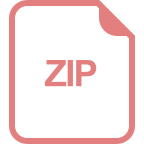
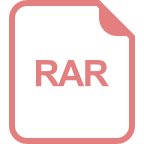
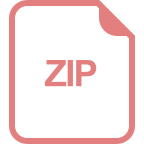
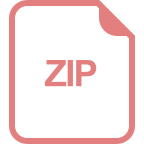
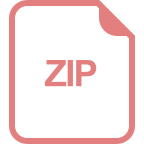
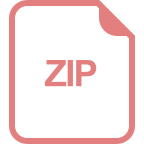
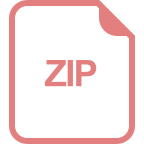
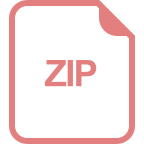
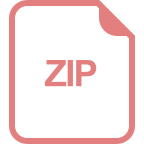
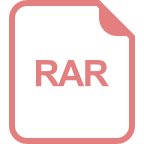
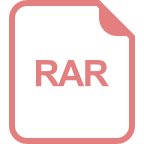
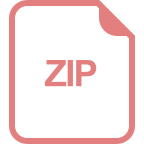
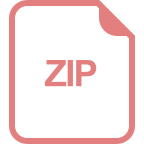
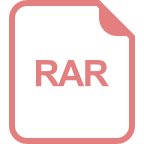
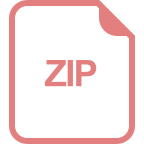