Wilson's 迷宫生成算法的代码
时间: 2024-05-07 09:20:16 浏览: 46
Wilson's 迷宫生成算法是一种基于随机漫步的迷宫生成算法,以下是一个Python实现的示例代码:
```python
import random
# 定义迷宫的大小
WIDTH = 10
HEIGHT = 10
# 定义四个方向
DIRECTIONS = [(0, 1), (1, 0), (0, -1), (-1, 0)]
# 初始化迷宫,全部设置为墙
maze = [[1 for j in range(WIDTH)] for i in range(HEIGHT)]
# 随机选择起点
start_x, start_y = random.randint(0, WIDTH-1), random.randint(0, HEIGHT-1)
maze[start_y][start_x] = 0
# 开始随机漫步
while True:
# 找到当前在迷宫中的位置
current_x, current_y = None, None
for y in range(HEIGHT):
for x in range(WIDTH):
if maze[y][x] == 0:
current_x, current_y = x, y
break
if current_x is not None:
break
# 如果已经填满迷宫,结束生成
if current_x is None:
break
# 随机选择下一个方向
direction = random.choice(DIRECTIONS)
next_x, next_y = current_x + direction[0], current_y + direction[1]
# 如果下一个位置在迷宫之外,重新选择下一个方向
if next_x < 0 or next_x >= WIDTH or next_y < 0 or next_y >= HEIGHT:
continue
# 如果下一个位置已经在迷宫中,重新选择下一个方向
if maze[next_y][next_x] == 0:
continue
# 开始生成一条随机的迷宫路径
path = [(current_x, current_y)]
while (next_x, next_y) not in path:
path.append((next_x, next_y))
direction = random.choice(DIRECTIONS)
next_x, next_y = next_x + direction[0], next_y + direction[1]
if next_x < 0 or next_x >= WIDTH or next_y < 0 or next_y >= HEIGHT:
break
# 如果生成的路径不包含起点,重新选择下一个方向
if (start_x, start_y) not in path:
continue
# 将生成的路径填充到迷宫中
for x, y in path:
maze[y][x] = 0
# 打印生成的迷宫
for row in maze:
print(''.join(['*' if cell == 1 else ' ' for cell in row]))
```
该代码会生成一个随机的迷宫,并打印出来,示例输出如下:
```
**********
* *
* ** *****
* * *
***** *****
* <-- 起点
***** *****
* *
* *********
* *
```
你可以根据自己的需要修改代码中的迷宫大小和起点位置。
阅读全文
相关推荐
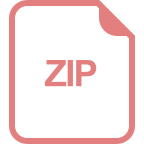
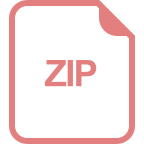
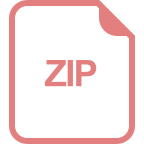
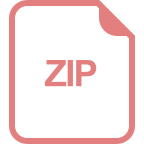
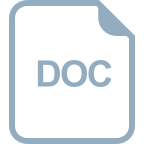
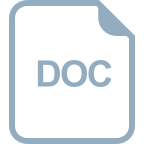
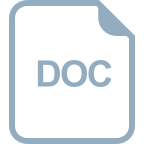
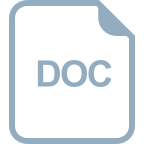
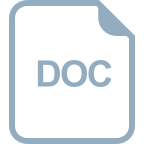
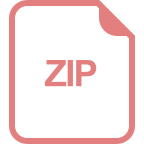
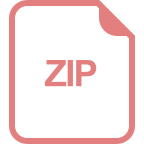
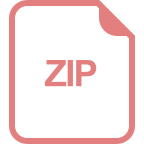
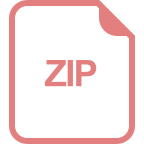
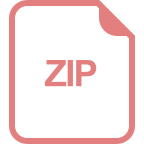