使用C语言写一个二叉排序树的插入算法函数,插入的变量为字符
时间: 2024-03-10 15:43:29 浏览: 61
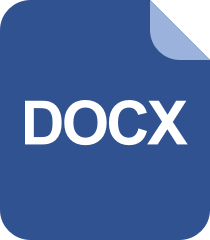
用C语言编写二叉排序树
好的,这是一个简单的二叉排序树的插入算法函数的实现,使用了递归方式:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉排序树结点的结构体
struct node {
char data;
struct node *left_child;
struct node *right_child;
};
// 插入函数
struct node* insert(struct node *root, char data) {
// 如果根结点为空,创建一个新结点并返回
if (root == NULL) {
struct node *new_node = (struct node*)malloc(sizeof(struct node));
new_node->data = data;
new_node->left_child = NULL;
new_node->right_child = NULL;
return new_node;
}
// 如果插入的值比根结点的值小,在左子树中插入
if (data < root->data) {
root->left_child = insert(root->left_child, data);
}
// 如果插入的值比根结点的值大,在右子树中插入
else if (data > root->data) {
root->right_child = insert(root->right_child, data);
}
// 如果插入的值等于根结点的值,返回根结点
else {
return root;
}
return root;
}
// 测试插入函数
int main() {
struct node *root = NULL;
root = insert(root, 'b');
insert(root, 'a');
insert(root, 'c');
insert(root, 'd');
insert(root, 'e');
insert(root, 'f');
insert(root, 'g');
printf("%c", root->left_child->right_child->right_child->data); // 输出 e
return 0;
}
```
以上代码中,我们定义了一个二叉排序树的结点结构体,包含了结点的值以及左右子结点的指针。然后定义了一个插入函数,使用递归方式在二叉排序树中插入新的结点。最后我们测试了插入函数,可以看到插入的字符按照二叉排序树的顺序排列,且插入正确。
阅读全文
相关推荐
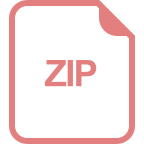
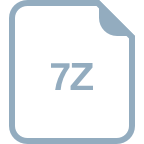
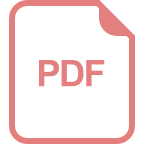
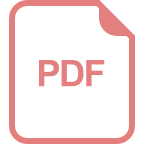
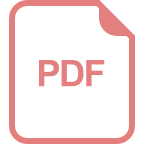
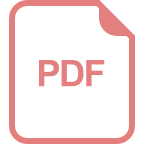
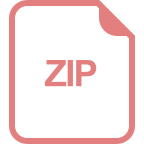
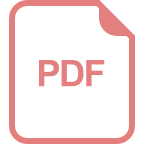
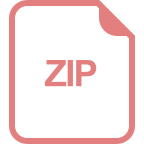
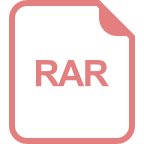
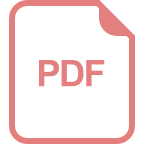
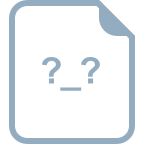
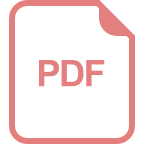
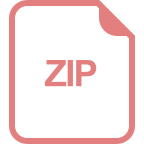
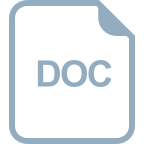
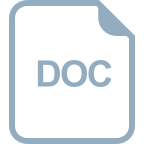