使用C语言编写一段遍历Linux/proc目录下所有文件的代码
时间: 2024-03-05 08:53:46 浏览: 103
下面是一个遍历Linux `/proc` 目录下所有文件的C语言代码:
```c
#include <stdio.h>
#include <dirent.h>
#include <string.h>
int main() {
DIR* dir = opendir("/proc"); // 打开目录
if (dir == NULL) {
perror("opendir failed");
return -1;
}
struct dirent* entry;
while ((entry = readdir(dir)) != NULL) { // 遍历目录
if (entry->d_type == DT_DIR && strcmp(entry->d_name, ".") != 0 && strcmp(entry->d_name, "..") != 0) {
// 过滤掉 "." 和 ".." 目录
printf("%s\n", entry->d_name);
}
}
closedir(dir); // 关闭目录
return 0;
}
```
此代码使用 `opendir` 打开 `/proc` 目录,然后使用 `readdir` 遍历目录中的所有文件。这里使用 `d_type` 属性过滤掉非目录文件,使用 `strcmp` 函数过滤掉 "." 和 ".." 目录,最后输出剩余的目录名字。
相关问题
使用C语言编写一段遍历Linux/proc目录下所有文件的代码,并判断文件名是否是数字
以下是使用C语言编写遍历Linux/proc目录下所有文件并判断文件名是否是数字的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <dirent.h>
#include <ctype.h>
int main() {
DIR *dir;
struct dirent *entry;
dir = opendir("/proc");
if (dir == NULL) {
perror("opendir");
exit(EXIT_FAILURE);
}
while ((entry = readdir(dir)) != NULL) {
if (isdigit(*entry->d_name)) {
printf("%s is a number\n", entry->d_name);
}
}
closedir(dir);
return 0;
}
```
注意:这个代码只是一个示例,可能会有一些问题,比如没有判断文件是否是目录等等。如果要在实际项目中使用,请根据实际情况进行修改和完善。
使用c语言编写一个读取 /proc/stat 文件中 每个cpu使用率的问题
在C语言中,要读取`/proc/stat`文件并计算每个CPU的使用率,你需要做以下几个步骤:
1. **打开文件**:首先,你需要使用`fopen()`函数打开`/proc/stat`文件,这个路径通常用于获取内核统计信息。
```c
#include <stdio.h>
FILE *fp;
fp = fopen("/proc/stat", "r");
```
2. **读取文件内容**:然后,你可以使用`fgets()`函数逐行读取文件,每行代表一个CPU的信息。
```c
char line[1024];
while (fgets(line, sizeof(line), fp) != NULL) {
// 对每一行处理...
}
```
3. **解析数据**:对于每一行,它通常是CPU状态信息的格式化字符串,例如`cpu 66917 2016 5985 1877 0 0 0 0 0 0`,需要分割成各个字段,并找到`cpu`对应的CPU使用时间(如`66917`)。这通常涉及到字符串处理和分割操作。
4. **计算CPU使用率**:CPU使用率可以用公式 `(系统时间 + 用户时间) / 总时间` 来计算,其中用户时间和系统时间是从`/proc/stat`文件中读取到的相应字段。将这些值转换为浮点数并计算百分比。
5. **处理和显示结果**:最后,对每个CPU的使用率进行适当的处理和显示。你可以选择直接打印出来,或者存储在一个结构体数组中供后续分析。
```c
double cpu_usage;
// 计算并存储CPU使用率
// ...
printf("CPU %d usage: %.2f%%\n", /* CPU编号 */, cpu_usage);
```
注意,实际的CPU计数可能会随硬件变化,所以你需要检查文件的前几行确定有多少个CPU。
阅读全文
相关推荐
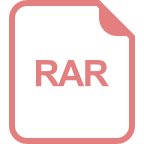
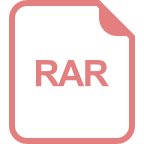
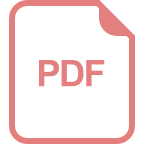












