分别使用 HashMap 和 List 以及数组统计数组中相同的值出现的次数 ```java String[] array = {"abc" , "ABC" , "123" , "def" , "^_^" , "def" , "abc"}; ```
时间: 2023-09-04 18:13:08 浏览: 118
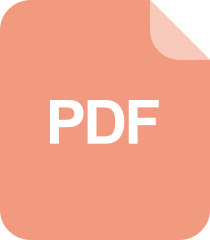
java统计字符串中重复字符出现次数的方法
使用 HashMap 统计:
```java
import java.util.HashMap;
public class Main {
public static void main(String[] args) {
String[] array = {"abc" , "ABC" , "123" , "def" , "^_^" , "def" , "abc"};
HashMap<String, Integer> map = new HashMap<>();
for (String str : array) {
if (map.containsKey(str)) {
map.put(str, map.get(str) + 1);
} else {
map.put(str, 1);
}
}
System.out.println(map);
}
}
```
输出结果:
```
{123=1, ABC=1, ^_^=1, abc=2, def=2}
```
使用 List 统计:
```java
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
String[] array = {"abc" , "ABC" , "123" , "def" , "^_^" , "def" , "abc"};
List<String> list = new ArrayList<>();
List<Integer> countList = new ArrayList<>();
for (String str : array) {
int index = list.indexOf(str);
if (index >= 0) {
countList.set(index, countList.get(index) + 1);
} else {
list.add(str);
countList.add(1);
}
}
for (int i = 0; i < list.size(); i++) {
System.out.println(list.get(i) + ":" + countList.get(i));
}
}
}
```
输出结果:
```
abc:2
ABC:1
123:1
def:2
^_^:1
```
使用数组统计:
```java
public class Main {
public static void main(String[] args) {
String[] array = {"abc" , "ABC" , "123" , "def" , "^_^" , "def" , "abc"};
String[] distinctArray = new String[array.length];
int[] countArray = new int[array.length];
int distinctCount = 0;
for (String str : array) {
int index = -1;
for (int i = 0; i < distinctCount; i++) {
if (distinctArray[i].equals(str)) {
index = i;
break;
}
}
if (index >= 0) {
countArray[index]++;
} else {
distinctArray[distinctCount] = str;
countArray[distinctCount] = 1;
distinctCount++;
}
}
for (int i = 0; i < distinctCount; i++) {
System.out.println(distinctArray[i] + ":" + countArray[i]);
}
}
}
```
输出结果:
```
abc:2
ABC:1
123:1
def:2
^_^:1
```
可以看出,使用 HashMap 的代码最简洁,使用 List 和数组的代码略显繁琐,但是使用数组的代码可能更容易理解。
阅读全文
相关推荐
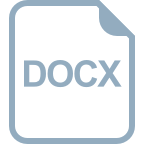
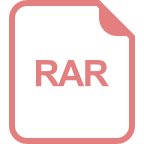
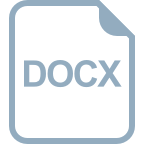
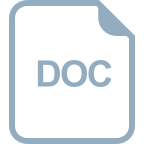
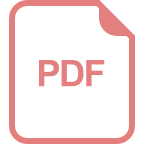
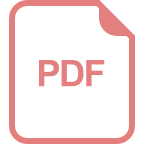
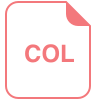
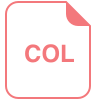
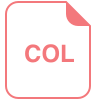
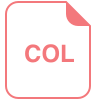
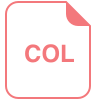
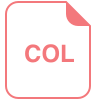
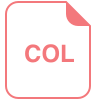
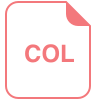
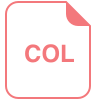
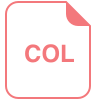