opencv python 颜色统计的代码实现
时间: 2023-08-04 21:17:56 浏览: 43
下面是使用OpenCV和Python实现颜色统计的代码示例:
```python
import cv2
import numpy as np
# 读取图像
img = cv2.imread('test.jpg')
# 转换颜色空间为HSV
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
# 定义蓝色的HSV范围
lower_blue = np.array([110, 50, 50])
upper_blue = np.array([130, 255, 255])
# 定义绿色的HSV范围
lower_green = np.array([50, 50, 50])
upper_green = np.array([70, 255, 255])
# 定义红色的HSV范围
lower_red = np.array([0, 50, 50])
upper_red = np.array([10, 255, 255])
lower_red2 = np.array([170, 50, 50])
upper_red2 = np.array([180, 255, 255])
# 提取蓝色区域
mask_blue = cv2.inRange(hsv, lower_blue, upper_blue)
res_blue = cv2.bitwise_and(img, img, mask=mask_blue)
blue_cnt = cv2.countNonZero(mask_blue)
# 提取绿色区域
mask_green = cv2.inRange(hsv, lower_green, upper_green)
res_green = cv2.bitwise_and(img, img, mask=mask_green)
green_cnt = cv2.countNonZero(mask_green)
# 提取红色区域
mask_red = cv2.inRange(hsv, lower_red, upper_red) + cv2.inRange(hsv, lower_red2, upper_red2)
res_red = cv2.bitwise_and(img, img, mask=mask_red)
red_cnt = cv2.countNonZero(mask_red)
# 显示结果
cv2.imshow('Original Image', img)
cv2.imshow('Blue', res_blue)
cv2.imshow('Green', res_green)
cv2.imshow('Red', res_red)
print('Blue pixels:', blue_cnt)
print('Green pixels:', green_cnt)
print('Red pixels:', red_cnt)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例中,我们首先读取图像,然后将其转换为HSV颜色空间。然后,我们定义了三种颜色(蓝色、绿色和红色)的HSV值范围。接下来,我们使用`cv2.inRange()`函数提取每种颜色的像素,并使用`cv2.bitwise_and()`函数将提取的像素与原始图像进行位运算,以获得颜色区域。最后,我们使用`cv2.countNonZero()`函数计算每种颜色的像素数量,并将其显示在屏幕上。
相关推荐
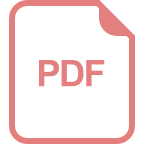














