c++ 使用openssl 1.1版本 实现rsa 1024加密
时间: 2024-02-18 14:00:24 浏览: 222
使用 OpenSSL 库可以更方便地实现 RSA 加密算法。以下是一个简单的示例程序,展示了如何使用 OpenSSL 库进行 RSA 1024 加密。
首先,需要安装 OpenSSL 库,并在程序中包含相应的头文件:
```c++
#include <openssl/rsa.h>
#include <openssl/pem.h>
```
接着,可以使用 OpenSSL 库中的函数生成 RSA 密钥对:
```c++
RSA *keypair = RSA_new();
BIGNUM *e = BN_new();
int bits = 1024;
unsigned long e_value = RSA_F4;
BN_set_word(e, e_value);
RSA_generate_key_ex(keypair, bits, e, NULL);
```
这里使用了 `RSA_new()` 函数创建了一个 RSA 结构体,使用 `BN_new()` 函数创建了一个大数结构体 `e`,表示公钥指数 `e` 的值,使用 `RSA_generate_key_ex()` 函数生成了 RSA 密钥对,其中 `bits` 表示密钥长度,`e` 表示公钥指数,`NULL` 表示使用默认的随机数生成器。
生成密钥对后,就可以使用公钥加密数据了。以下是一个示例函数,用于 RSA 加密:
```c++
// 使用公钥加密数据
// 返回加密后的数据长度,加密失败返回0
int rsa_encrypt(const unsigned char *src, int src_len,
unsigned char *dst, RSA *key) {
int flen = RSA_size(key);
int ret = RSA_public_encrypt(src_len, src, dst, key, RSA_PKCS1_PADDING);
if (ret != flen) {
return 0;
}
return ret;
}
```
其中,`src` 表示要加密的数据,`src_len` 表示数据长度,`dst` 表示加密后的数据,`key` 表示公钥。
接着,可以使用私钥解密数据。以下是一个示例函数,用于 RSA 解密:
```c++
// 使用私钥解密数据
// 返回解密后的数据长度,解密失败返回0
int rsa_decrypt(const unsigned char *src, int src_len,
unsigned char *dst, RSA *key) {
int flen = RSA_size(key);
int ret = RSA_private_decrypt(src_len, src, dst, key, RSA_PKCS1_PADDING);
if (ret <= 0 || ret >= flen) {
return 0;
}
return ret;
}
```
其中,`src` 表示要解密的数据,`src_len` 表示数据长度,`dst` 表示解密后的数据,`key` 表示私钥。
完整的示例程序如下:
```c++
#include <iostream>
#include <cstring>
#include <openssl/rsa.h>
#include <openssl/pem.h>
using namespace std;
// 使用公钥加密数据
// 返回加密后的数据长度,加密失败返回0
int rsa_encrypt(const unsigned char *src, int src_len,
unsigned char *dst, RSA *key) {
int flen = RSA_size(key);
int ret = RSA_public_encrypt(src_len, src, dst, key, RSA_PKCS1_PADDING);
if (ret != flen) {
return 0;
}
return ret;
}
// 使用私钥解密数据
// 返回解密后的数据长度,解密失败返回0
int rsa_decrypt(const unsigned char *src, int src_len,
unsigned char *dst, RSA *key) {
int flen = RSA_size(key);
int ret = RSA_private_decrypt(src_len, src, dst, key, RSA_PKCS1_PADDING);
if (ret <= 0 || ret >= flen) {
return 0;
}
return ret;
}
int main() {
RSA *keypair = RSA_new();
BIGNUM *e = BN_new();
int bits = 1024;
unsigned long e_value = RSA_F4;
BN_set_word(e, e_value);
RSA_generate_key_ex(keypair, bits, e, NULL);
// 输出公钥和私钥
BIO *bp_private = BIO_new(BIO_s_mem());
BIO *bp_public = BIO_new(BIO_s_mem());
PEM_write_bio_RSAPrivateKey(bp_private, keypair, NULL, NULL, 0, NULL, NULL);
PEM_write_bio_RSAPublicKey(bp_public, keypair);
char *private_key;
char *public_key;
long private_key_len = BIO_get_mem_data(bp_private, &private_key);
long public_key_len = BIO_get_mem_data(bp_public, &public_key);
cout << "Private Key: " << endl << string(private_key, private_key_len) << endl;
cout << "Public Key: " << endl << string(public_key, public_key_len) << endl;
// 加密
const unsigned char *msg = (const unsigned char *)"Hello, world!";
int msg_len = strlen((const char *)msg);
unsigned char ciphertext[4096];
int ciphertext_len = rsa_encrypt(msg, msg_len, ciphertext, keypair);
cout << "Encrypted Message: " << endl;
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
cout << endl;
// 解密
unsigned char plaintext[4096];
int plaintext_len = rsa_decrypt(ciphertext, ciphertext_len, plaintext, keypair);
cout << "Decrypted Message: " << endl;
cout << string((const char *)plaintext, plaintext_len) << endl;
RSA_free(keypair);
BN_free(e);
BIO_free_all(bp_private);
BIO_free_all(bp_public);
return 0;
}
```
该程序使用 OpenSSL 库生成 RSA 密钥对,并使用公钥加密和私钥解密数据。注意,为了方便输出公钥和私钥,该程序使用了 `PEM_write_bio_RSAPrivateKey()` 和 `PEM_write_bio_RSAPublicKey()` 函数将密钥转换成 PEM 格式输出。在实际应用中,可以直接使用 RSA 结构体表示密钥。
阅读全文
相关推荐
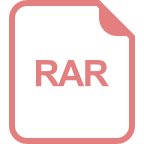
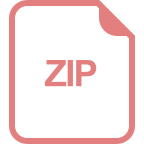
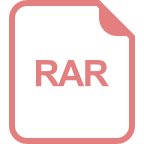
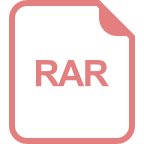
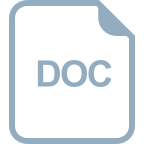




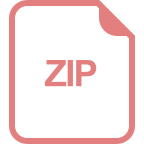
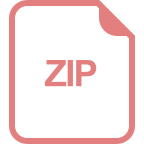
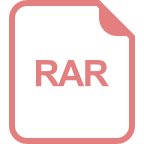
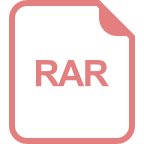
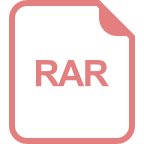
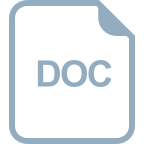
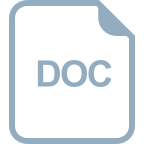