java 链接生成二维码
时间: 2023-09-01 15:07:15 浏览: 84
以下是使用zxing库生成二维码的Java代码示例:
```java
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
public class QRCodeGenerator {
private static final String CHARSET = "UTF-8";
private static final int QRCODE_SIZE = 300;
public static void main(String[] args) {
String url = "https://www.example.com/";
try {
BufferedImage image = generateQRCodeImage(url);
ImageIO.write(image, "png", new File("qrcode.png"));
System.out.println("QR code image created successfully!");
} catch (IOException e) {
System.out.println("Could not create QR code image: " + e.getMessage());
}
}
private static BufferedImage generateQRCodeImage(String text) throws WriterException, IOException {
Map<EncodeHintType, Object> hintMap = new HashMap<>();
hintMap.put(EncodeHintType.ERROR_CORRECTION, com.google.zxing.qrcode.decoder.ErrorCorrectionLevel.L);
hintMap.put(EncodeHintType.CHARACTER_SET, CHARSET);
hintMap.put(EncodeHintType.MARGIN, 1);
QRCodeWriter qrCodeWriter = new QRCodeWriter();
BitMatrix bitMatrix = qrCodeWriter.encode(text, BarcodeFormat.QR_CODE, QRCODE_SIZE, QRCODE_SIZE, hintMap);
int width = bitMatrix.getWidth();
BufferedImage image = new BufferedImage(width, width, BufferedImage.TYPE_INT_RGB);
int white = 255 << 16 | 255 << 8 | 255;
int black = 0;
for (int x = 0; x < width; x++) {
for (int y = 0; y < width; y++) {
image.setRGB(x, y, bitMatrix.get(x, y) ? black : white);
}
}
return image;
}
}
```
在上面的代码中,我们首先定义了字符集和二维码尺寸。然后,我们使用zxing库创建了一个QRCodeWriter实例,并向其传递一些编码提示,例如错误纠正级别、字符集和边缘大小。接下来,我们使用QRCodeWriter实例的encode()方法来生成一个BitMatrix对象,该对象包含二维码的位矩阵表示。最后,我们遍历位矩阵并将其转换为BufferedImage对象,然后将其保存为PNG文件。
要生成包含链接的二维码,只需将链接传递给generateQRCodeImage()方法即可。例如,如果要生成包含"https://www.example.com/"链接的二维码,只需调用generateQRCodeImage("https://www.example.com/")。
阅读全文
相关推荐

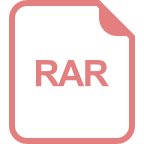
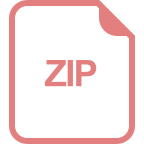
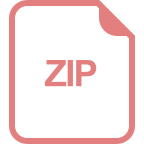
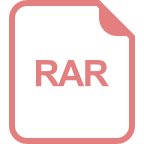
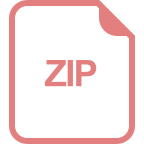
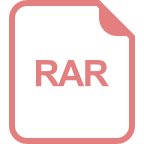
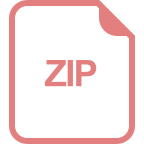
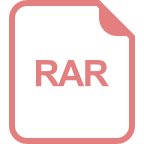
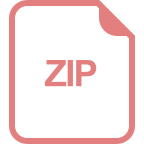
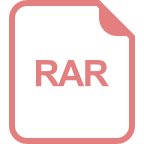
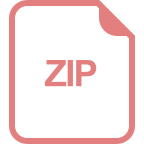
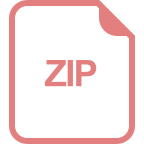
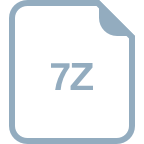
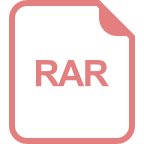