c语言递归解二元不定方程组
时间: 2023-06-21 15:13:21 浏览: 191
递归求解二元不定方程组的方法如下:
首先,我们可以将一个二元不定方程组表示为以下形式:
ax + by = c
其中,a、b、c为已知常数,x、y为未知数。
我们需要先将方程组化简为最简形式,例如消元法将方程组化简为以下形式:
ax + by = c
dx + ey = f
其中,a、b、c、d、e、f为已知常数,x、y为未知数。
然后,我们可以使用递归的方式求解该方程组。具体地,我们可以将该方程组化为以下形式:
x = (c - by) / a
y = (f - dx) / e
然后,我们可以将x、y代入原方程组中,检验解是否正确。如果解不正确,我们可以将该方程组继续化简,直到求出正确的解为止。
下面是一个递归求解二元不定方程组的示例代码:
```
#include <stdio.h>
int solve_eq(int a, int b, int c, int d, int e, int f, int *x, int *y)
{
int det = a * e - b * d;
if (det == 0) {
return 0;
}
int x1 = (c * e - b * f) / det;
int y1 = (a * f - c * d) / det;
if (a * x1 + b * y1 != c || d * x1 + e * y1 != f) {
return 0;
}
*x = x1;
*y = y1;
return 1;
}
int main()
{
int a, b, c, d, e, f, x, y;
printf("Enter the coefficients of the equation:\n");
scanf("%d %d %d %d %d %d", &a, &b, &c, &d, &e, &f);
if (solve_eq(a, b, c, d, e, f, &x, &y)) {
printf("The solution is: x=%d, y=%d\n", x, y);
} else {
printf("No solution exists\n");
}
return 0;
}
```
注意,递归求解二元不定方程组的时间复杂度是指数级别的,因此对于较大的方程组,该方法可能会非常慢。
阅读全文
相关推荐

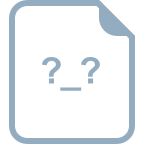
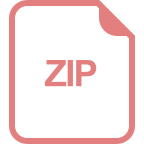
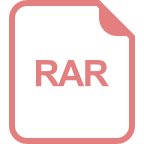
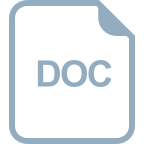
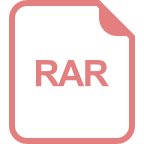
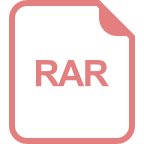
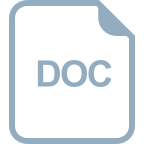
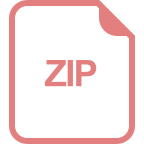