C语言使用定时器和线程完成贪吃蛇并且写出源代码
时间: 2024-02-23 08:06:22 浏览: 44
好的,以下是使用C语言实现贪吃蛇游戏的源代码,其中使用了定时器和线程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 60
#define HEIGHT 30
#define SNAKE_MAX_LENGTH 100
int map[HEIGHT][WIDTH]; // 地图,0表示空格,1表示蛇身,2表示食物
int snake_x[SNAKE_MAX_LENGTH], snake_y[SNAKE_MAX_LENGTH]; // 蛇的坐标
int snake_length; // 蛇的长度
int food_x, food_y; // 食物的坐标
int direction; // 蛇的移动方向,0表示上,1表示右,2表示下,3表示左
// 初始化游戏
void init_game() {
int i, j;
// 初始化地图
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
map[i][j] = 0;
}
}
// 初始化蛇
snake_x[0] = WIDTH / 2;
snake_y[0] = HEIGHT / 2;
snake_length = 1;
map[snake_y[0]][snake_x[0]] = 1;
// 初始化食物
srand((unsigned)time(NULL));
food_x = rand() % WIDTH;
food_y = rand() % HEIGHT;
map[food_y][food_x] = 2;
// 初始化方向
direction = 0;
}
// 绘制地图
void draw_map() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
if (map[i][j] == 0) {
printf(" ");
} else if (map[i][j] == 1) {
printf("*");
} else if (map[i][j] == 2) {
printf("O");
}
}
printf("\n");
}
}
// 蛇的移动
void move_snake() {
int i;
// 计算蛇头的新位置
int new_x = snake_x[0], new_y = snake_y[0];
if (direction == 0) {
new_y--;
} else if (direction == 1) {
new_x++;
} else if (direction == 2) {
new_y++;
} else {
new_x--;
}
// 判断是否撞墙或撞到自己
if (new_x < 0 || new_x >= WIDTH || new_y < 0 || new_y >= HEIGHT || map[new_y][new_x] == 1) {
printf("Game over!\n");
exit(0);
}
// 判断是否吃到食物
if (new_x == food_x && new_y == food_y) {
snake_length++;
food_x = rand() % WIDTH;
food_y = rand() % HEIGHT;
map[food_y][food_x] = 2;
} else {
map[snake_y[snake_length - 1]][snake_x[snake_length - 1]] = 0;
}
// 更新蛇的位置
for (i = snake_length - 1; i > 0; i--) {
snake_x[i] = snake_x[i - 1];
snake_y[i] = snake_y[i - 1];
}
snake_x[0] = new_x;
snake_y[0] = new_y;
map[new_y][new_x] = 1;
}
// 用户输入线程
DWORD WINAPI input_thread(LPVOID lpParam) {
while (1) {
int key = getch();
if (key == 72) {
direction = 0;
} else if (key == 77) {
direction = 1;
} else if (key == 80) {
direction = 2;
} else if (key == 75) {
direction = 3;
}
}
}
// 定时器回调函数
void CALLBACK timer_callback(HWND hwnd, UINT uMsg, UINT_PTR idEvent, DWORD dwTime) {
move_snake();
system("cls");
draw_map();
}
// 主函数
int main() {
init_game();
draw_map();
// 创建用户输入线程
HANDLE hThread = CreateThread(NULL, 0, input_thread, NULL, 0, NULL);
if (hThread == NULL) {
printf("CreateThread failed!\n");
return 1;
}
// 创建定时器
UINT_PTR timer_id = SetTimer(NULL, 0, 200, timer_callback);
if (timer_id == 0) {
printf("SetTimer failed!\n");
return 1;
}
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// 销毁定时器
KillTimer(NULL, timer_id);
return 0;
}
```
以上代码使用了Windows API中的定时器和线程函数来实现。在主函数中,我们首先调用init_game函数来初始化游戏,然后调用draw_map函数来绘制地图。接着,我们创建一个用户输入线程,并且创建一个定时器,回调函数为timer_callback。在回调函数中,我们调用move_snake函数来移动蛇,并且绘制地图。最后,在消息循环中,我们调用GetMessage函数来等待消息,当收到WM_QUIT消息时,程序退出,并且销毁定时器。
相关推荐
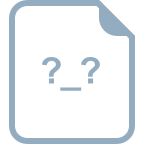














