基于winpcap的网络抓包python程序
时间: 2023-12-20 12:04:15 浏览: 240
以下是一个基于WinPcap的网络抓包Python程序的示例代码:
```
import socket
import struct
import sys
import threading
from ctypes import *
from winpcapy import *
# define constants
PCAP_ERRBUF_SIZE = 256
# define structure for IP header
class IP(Structure):
_fields_ = [
("ihl", c_ubyte, 4),
("version", c_ubyte, 4),
("tos", c_ubyte),
("len", c_ushort),
("id", c_ushort),
("offset", c_ushort),
("ttl", c_ubyte),
("protocol_num", c_ubyte),
("sum", c_ushort),
("src", c_ulong),
("dst", c_ulong)
]
def __new__(self, data=None):
return self.from_buffer_copy(data)
def __init__(self, data=None):
# map protocol constants to their names
self.protocol_map = {1: "ICMP", 6: "TCP", 17: "UDP"}
# human readable IP addresses
self.src_address = socket.inet_ntoa(struct.pack("<L", self.src))
self.dst_address = socket.inet_ntoa(struct.pack("<L", self.dst))
# human readable protocol
try:
self.protocol = self.protocol_map[self.protocol_num]
except:
self.protocol = str(self.protocol_num)
# define callback function for packet capture
def packet_handler(header, data):
# parse IP header
ip_header = IP(data)
# print out packet information
print("Protocol: %s, Source: %s, Destination: %s" % (ip_header.protocol, ip_header.src_address, ip_header.dst_address))
def main():
# open network adapter for capturing
errbuf = create_string_buffer(PCAP_ERRBUF_SIZE)
adapter = pcap_open_live("eth0", 65536, 1, 1000, errbuf)
if not adapter:
print("Unable to open adapter: %s" % errbuf.value.decode("utf-8"))
sys.exit(1)
# start packet capture loop
try:
pcap_loop(adapter, -1, packet_handler, None)
except KeyboardInterrupt:
pass
# close the adapter
pcap_close(adapter)
if __name__ == "__main__":
main()
```
这个程序使用WinPcap库来捕获网络数据包,并使用Python中的ctypes库来定义IP头的结构体。程序打开一个名为“eth0”的网络适配器,然后进入无限循环以捕获数据包。当用户按下Ctrl + C时,程序将退出循环并关闭适配器。程序还包括一个包处理程序回调函数,它将在每个捕获的数据包上运行,并打印有关该包的一些信息。
阅读全文
相关推荐
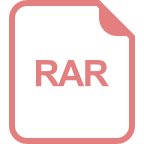


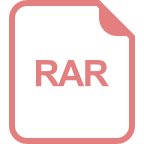
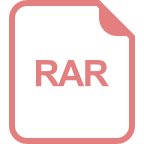









