java 解决base64长度过长问题
时间: 2023-08-18 11:06:56 浏览: 592
Base64编码是将任意二进制数据转换成纯文本格式的编码方式,但是在某些情况下,经过Base64编码后的字符串长度可能会超过所允许的最大长度限制,例如在HTTP请求头部中使用Base64编码时。
解决这个问题的方法是将超长的Base64字符串分割成多个片段进行传输,这样可以保证每个片段的长度不会超过长度限制。在Java中,可以使用以下代码将Base64字符串进行分割:
```java
public static String[] splitBase64String(String base64String, int splitLength) {
if (base64String == null || base64String.isEmpty()) {
return new String[]{};
}
if (splitLength <= 0) {
throw new IllegalArgumentException("splitLength must be greater than 0");
}
byte[] bytes = Base64.getDecoder().decode(base64String);
List<String> stringList = new ArrayList<>();
int totalLength = bytes.length;
int currentIndex = 0;
while (currentIndex < totalLength) {
int endIndex = Math.min(currentIndex + splitLength, totalLength);
byte[] subBytes = Arrays.copyOfRange(bytes, currentIndex, endIndex);
String subString = Base64.getEncoder().encodeToString(subBytes);
stringList.add(subString);
currentIndex = endIndex;
}
return stringList.toArray(new String[stringList.size()]);
}
```
该方法接受两个参数,第一个参数是需要分割的Base64字符串,第二个参数是每个分割片段的最大长度。该方法会将Base64字符串先解码成字节数组,然后将字节数组分割成多个片段,并将每个片段重新编码成Base64字符串返回。
使用该方法的示例代码:
```java
String base64String = "aGVsbG8gd29ybGQ=";
String[] base64Strings = splitBase64String(base64String, 4);
for (String str : base64Strings) {
System.out.println(str);
}
```
输出结果:
```
aGVs
bG8g
d29y
bGQ=
```
在实际应用中,可以根据需要调整分割片段的最大长度,以达到最佳的传输效率。
阅读全文
相关推荐
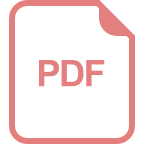
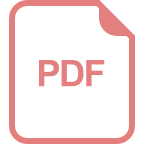
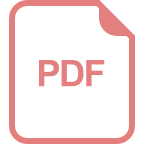






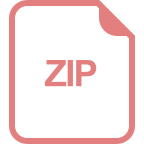
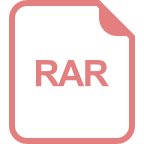
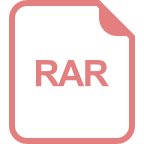
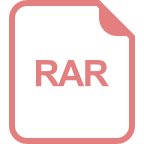
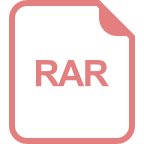
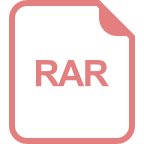
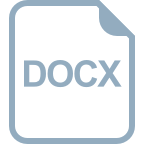
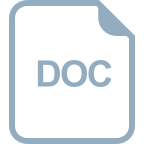
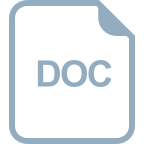

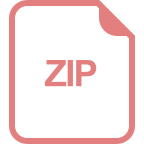