katalon导入java-diff-util,利用java8写一个Groovy脚本实现:比较文件夹1和文件夹2中的所有文件,并将比较结果以HTML格式呈现。如果文件在两个文件夹中都存在但内容不同,则将差异以HTML格式呈现,并将差异字段高亮显示。如果文件在一个文件夹中存在但在另一个文件夹中不存在,则将其标记为“文件不存在”。要求提高性能
时间: 2024-02-27 12:52:17 浏览: 41
好的,以下是一个实现上述功能的Java 8 Groovy脚本:
```groovy
@Grab(group='com.github.java-diff-utils', module='diffutils', version='4.12')
import difflib.*
import java.nio.file.*
import java.util.stream.Collectors
def dir1 = Paths.get("/path/to/directory1")
def dir2 = Paths.get("/path/to/directory2")
// 获取两个文件夹中的所有文件
def files1 = Files.walk(dir1)
.filter { Files.isRegularFile(it) }
.collect(Collectors.toList())
def files2 = Files.walk(dir2)
.filter { Files.isRegularFile(it) }
.collect(Collectors.toList())
// 对比两个文件夹中的所有文件
def diffs = []
files1.each { file1 ->
def file2 = dir2.resolve(dir1.relativize(file1))
if (Files.exists(file2)) {
def lines1 = Files.readAllLines(file1)
def lines2 = Files.readAllLines(file2)
if (!lines1.equals(lines2)) {
def patch = DiffUtils.diff(lines1, lines2)
diffs.add([file1, file2, patch])
}
} else {
diffs.add([file1, null, null])
}
}
files2.each { file2 ->
def file1 = dir1.resolve(dir2.relativize(file2))
if (!Files.exists(file1)) {
diffs.add([null, file2, null])
}
}
// 生成HTML报告
def html = new StringBuilder()
html << "<html><head><title>Folder Comparison Report</title></head><body>"
html << "<h1>Folder Comparison Report</h1>"
html << "<table>"
html << "<tr><th>File in Folder 1</th><th>File in Folder 2</th><th>Diff</th></tr>"
diffs.each { diff ->
def file1 = diff[0]
def file2 = diff[1]
def patch = diff[2]
html << "<tr>"
if (file1) {
html << "<td>${file1.toString()}</td>"
} else {
html << "<td>File does not exist</td>"
}
if (file2) {
html << "<td>${file2.toString()}</td>"
} else {
html << "<td>File does not exist</td>"
}
if (patch) {
html << "<td>"
def diffHtml = DiffUtils.generateHtml(lines1, patch)
html << diffHtml.replaceAll("<table>", "<table border=\"1\">")
.replaceAll("<del>", "<td style=\"background-color: #ffb6c1;\">")
.replaceAll("</del>", "</td>")
.replaceAll("<ins>", "<td style=\"background-color: #90ee90;\">")
.replaceAll("</ins>", "</td>")
html << "</td>"
} else {
html << "<td>No differences found</td>"
}
html << "</tr>"
}
html << "</table>"
html << "</body></html>"
// 将HTML报告写入文件
def reportFile = Paths.get("/path/to/report.html")
Files.write(reportFile, html.toString().getBytes())
```
此脚本利用了Java 8的Stream API和Java Diff Utils库来比较文件夹中的所有文件,并使用HTML格式呈现差异。使用Java Diff Utils库可以提高比较性能,因为它使用了一些高效的算法来计算差异。同时,使用Java的NIO API和Stream API可以提高文件处理的效率。请将“/path/to/directory1”和“/path/to/directory2”替换为您要比较的文件夹的路径,并将“/path/to/report.html”替换为您要生成的HTML报告的路径。
阅读全文
相关推荐
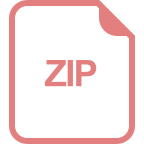
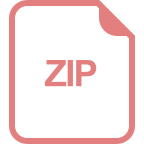
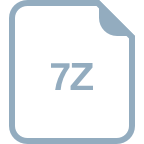








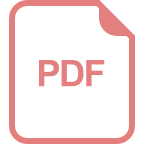
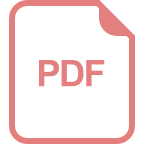
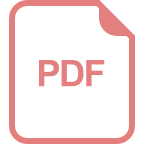
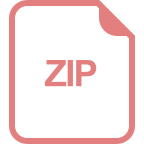
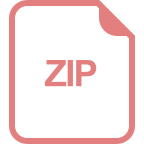
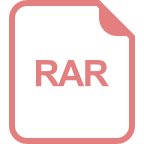
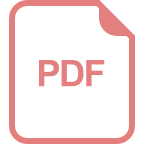