throttle-debounce使用方法
时间: 2023-09-14 22:12:13 浏览: 101
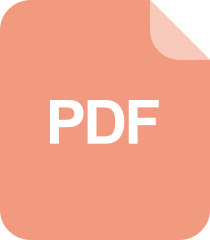
vue以组件或者插件的形式实现throttle或者debounce
throttle和debounce都是用于控制函数的执行频率的方法。
throttle的作用是在一段时间内只执行一次函数,比如一个按钮的点击事件,在用户多次点击时只执行一次,以免出现重复操作的情况。
debounce的作用是在一段时间内不再触发函数后再执行函数,比如用户在输入框中输入文字时,只有在停止输入一段时间后才会执行搜索操作,以免频繁触发搜索操作。
下面是throttle和debounce的使用方法:
Throttle:
```javascript
function throttle(func, delay) {
let prev = Date.now() - delay;
return function() {
const context = this;
const args = arguments;
const now = Date.now();
if (now - prev >= delay) {
func.apply(context, args);
prev = now;
}
}
}
```
使用方法:
```javascript
function handleClick() {
console.log('clicked');
}
const throttledClick = throttle(handleClick, 1000);
button.addEventListener('click', throttledClick);
```
Debounce:
```javascript
function debounce(func, delay) {
let timer;
return function() {
const context = this;
const args = arguments;
clearTimeout(timer);
timer = setTimeout(function() {
func.apply(context, args);
}, delay);
}
}
```
使用方法:
```javascript
function handleInput() {
console.log('searching');
}
const debouncedInput = debounce(handleInput, 500);
input.addEventListener('input', debouncedInput);
```
以上代码是纯JavaScript实现的throttle和debounce,当然也可以使用一些第三方库来实现,比如lodash的throttle和debounce函数。
阅读全文
相关推荐
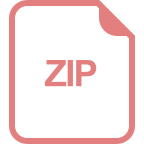
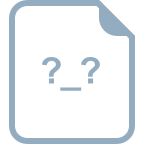
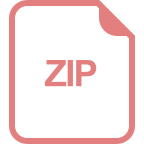
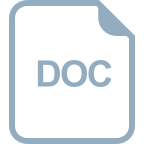



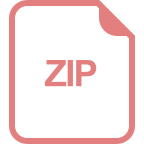
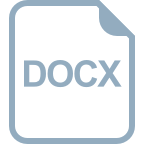
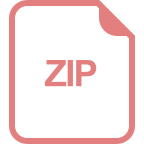
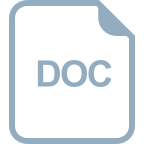
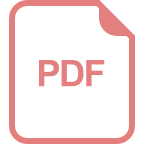
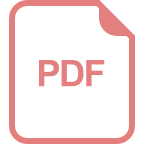
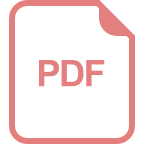
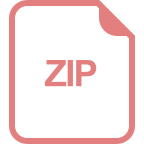