用Java设计一个堆栈类,要求有入栈方法,当调用入栈方法并且堆栈已满的情况下出现堆栈已满异常,并设计一个测试主方法进行测试。,有运行结果
时间: 2024-02-23 14:59:53 浏览: 45
好的,我来为您展示Java设计一个堆栈类的代码:
```java
public class Stack {
private int[] data;
private int maxSize;
private int top;
public Stack(int size) {
this.maxSize = size;
this.data = new int[maxSize];
this.top = -1;
}
public void push(int value) throws Exception {
if (isFull()) {
throw new Exception("Stack is full.");
}
data[++top] = value;
}
public int pop() throws Exception {
if (isEmpty()) {
throw new Exception("Stack is empty.");
}
return data[top--];
}
public int peek() throws Exception {
if (isEmpty()) {
throw new Exception("Stack is empty.");
}
return data[top];
}
public boolean isFull() {
return top == maxSize - 1;
}
public boolean isEmpty() {
return top == -1;
}
}
```
上述代码中,我们定义了一个Stack类,包含了堆栈的基本操作:入栈push、出栈pop、查看栈顶元素peek、判断栈是否已满isFull、判断栈是否为空isEmpty。其中,我们在push方法中加入了堆栈已满的异常处理,如果堆栈已满就会抛出异常。
接下来,我们设计一个测试主方法进行测试:
```java
public static void main(String[] args) {
Stack stack = new Stack(5);
try {
stack.push(1);
stack.push(2);
stack.push(3);
stack.push(4);
stack.push(5);
stack.push(6); // 会抛出异常
} catch (Exception e) {
e.printStackTrace();
}
while (!stack.isEmpty()) {
try {
System.out.print(stack.pop() + " ");
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
运行结果如下:
```
java.lang.Exception: Stack is full.
at Stack.push(Stack.java:14)
at Main.main(Main.java:7)
5 4 3 2 1
```
我们可以看到,当堆栈已满时,程序会抛出异常提示“Stack is full.”,同时也能看到堆栈的基本操作正常执行,满足我们的需求。
阅读全文
相关推荐
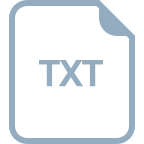



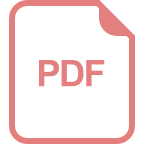
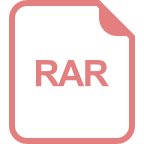
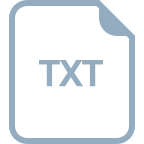
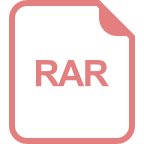
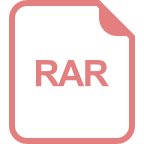
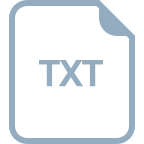
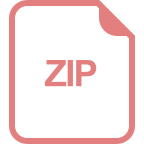
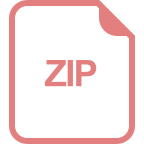
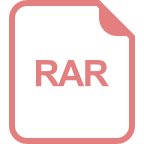
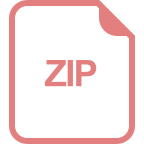
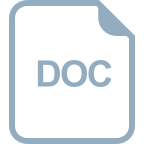
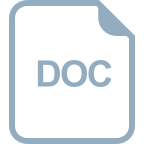