java得到控制台输入的年月日(yyyy,m,d)
时间: 2024-03-21 13:37:38 浏览: 21
您可以使用Java中的Scanner类来获取控制台输入的年月日。以下是一个示例代码:
```java
import java.util.Scanner;
public class ConsoleInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入年份:");
int year = scanner.nextInt();
System.out.print("请输入月份:");
int month = scanner.nextInt();
System.out.print("请输入日期:");
int day = scanner.nextInt();
System.out.println("输入的日期为:" + year + "-" + month + "-" + day);
scanner.close();
}
}
```
在这个示例中,我们首先创建一个Scanner对象来读取控制台输入。然后,我们使用nextInt()方法分别读取年、月、日的输入,并将它们存储在相应的整数变量中。最后,我们将这些变量组合成一个字符串并输出到控制台上。
注意,在使用Scanner类之后,我们需要调用其close()方法来释放相关资源。
相关问题
java 格式化日期 年月日
在Java中,可以使用SimpleDateFormat类来格式化日期。以下是一个示例代码,演示如何格式化日期为年月日的格式:
```java
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateFormatExample {
public static main(String[] args) // 创建一个SimpleDateFormat对象,指定日期格式
SimpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");
// 获取当前日期
Date currentDate = new Date();
使用SimpleDateFormat对象将日期格式化为指定格式的字符串
String formattedDate = sdf.format(currentDate);
// 输出格式化后的日期字符串
System.out.println("Formatted Date: " + formattedDate);
}
}
```
在上面的示例中,我们创建了一个SimpleDateFormat对象,并通过构造函数传入日期格式"yyyy-MM-dd",表示年月日的格式。然后,我们使用format()方法将当前日期格式化为指定格式的字符串。最后,我们将格式化后的日期字符串输出到控制台。
你也可以根据需要自定义日期格式,例如"MM/dd/yyyy"表示月日年的格式,"dd/MM/yyyy"表示日月年的格式等。只需将对应的日期格式传递给SimpleDateFormat的构造函数即可。
使用java实现年月日日期查询
### 回答1:
在Java中可以使用 `java.util.Calendar` 类来实现年月日日期查询。
你可以使用 `Calendar.getInstance()` 方法获取当前日期,然后使用以下方法来获取年份、月份、日期:
```
Calendar calendar = Calendar.getInstance();
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH) + 1; // 月份从0开始,所以要加1
int day = calendar.get(Calendar.DAY_OF_MONTH);
```
如果你想查询某个特定日期,可以使用 `Calendar.set` 方法设置日期,然后使用上面的方法获取年月日:
```
Calendar calendar = Calendar.getInstance();
calendar.set(2022, 2, 1); // 2022年3月1日
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH) + 1;
int day = calendar.get(Calendar.DAY_OF_MONTH);
```
需要注意的是,月份在 `Calendar` 中是从0开始的,所以3月对应的数字是2。
### 回答2:
使用Java实现年月日日期查询可以通过使用Java中的日期类库来实现。以下是一个简单的示例代码:
```java
import java.time.LocalDate;
public class DateQuery {
public static void main(String[] args) {
// 获取当前日期
LocalDate currentDate = LocalDate.now();
// 打印当前日期
System.out.println("当前日期:" + currentDate);
// 查询指定年份是否是闰年
int year = 2022;
boolean isLeapYear = currentDate.isLeapYear();
System.out.println(year + "年是否是闰年:" + isLeapYear);
// 查询指定日期是星期几
LocalDate date = LocalDate.of(2022, 1, 1);
String dayOfWeek = date.getDayOfWeek().toString();
System.out.println("2022年1月1日是星期:" + dayOfWeek);
}
}
```
该代码中,我们首先使用`LocalDate.now()`方法获取当前日期,并打印出来。然后,我们查询指定年份是否是闰年,使用的是`isLeapYear()`方法,该方法返回一个布尔值来表示是否是闰年。最后,我们查询指定日期是星期几,使用的是`getDayOfWeek()`方法,该方法返回一个`DayOfWeek`枚举类型来表示星期几,我们可以通过调用`toString()`方法将其转换为具体的星期几的字符串。
当然,这只是一个简单的示例,你可以根据具体需求进行更复杂的年月日日期查询的实现。
### 回答3:
使用Java实现年月日日期查询可以通过使用Java的日期时间类库来实现。
首先,我们需要导入java.time包中的相关类来处理日期操作。这些类包括LocalDate、LocalDateTime、DateTimeFormatter等。
首先,我们创建一个Scanner对象,用于从控制台接收用户输入的日期信息。
然后,我们使用Scanner对象接收用户输入的日期信息,并将其保存在一个String变量中。
接着,我们使用DateTimeFormatter类来定义日期的格式。格式包括年份(yyyy)、月份(MM)和日期(dd)。
然后,我们使用LocalDate类的parse方法将用户输入的日期字符串转换为LocalDate对象。
接下来,我们可以通过LocalDate对象的getYear、getMonthValue和getDayOfMonth方法获取到年、月和日的具体数值。
最后,我们可以将获取到的年、月、日数值输出到控制台,以完成日期查询的功能。
以下是示例代码:
```java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.Scanner;
public class DateQuery {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入日期(格式:yyyy-MM-dd):");
String dateString = scanner.next();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
LocalDate date = LocalDate.parse(dateString, formatter);
int year = date.getYear();
int month = date.getMonthValue();
int day = date.getDayOfMonth();
System.out.println("年: " + year);
System.out.println("月: " + month);
System.out.println("日: " + day);
}
}
```
以上就是使用Java实现年月日日期查询的方法。
相关推荐
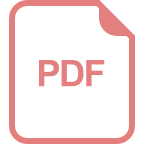
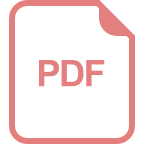
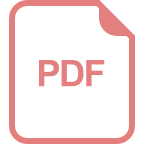
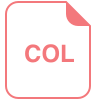
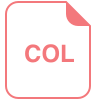
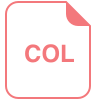
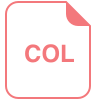








