在Linux环境用C语言编写文件复制程序。分别使用系统调用read (fd,buf,nbytes)、write(fd, buf,nbytes)和文件的库函数fread(buf,size,nitems,fp)、fwrite(buf,size,nitems,fp),编写一个文件复制程序
时间: 2024-02-03 11:14:42 浏览: 115
下面是使用系统调用read和write函数实现的文件复制程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
if (argc != 3) {
fprintf(stderr, "Usage: %s source_file dest_file\n", argv[0]);
exit(EXIT_FAILURE);
}
int source_fd = open(argv[1], O_RDONLY);
if (source_fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
int dest_fd = open(argv[2], O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (dest_fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
char buffer[BUFFER_SIZE];
ssize_t read_count, write_count;
while ((read_count = read(source_fd, buffer, BUFFER_SIZE)) > 0) {
write_count = write(dest_fd, buffer, read_count);
if (write_count == -1) {
perror("write");
exit(EXIT_FAILURE);
}
}
if (read_count == -1) {
perror("read");
exit(EXIT_FAILURE);
}
if (close(source_fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
if (close(dest_fd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
return 0;
}
```
下面是使用文件的库函数fread和fwrite函数实现的文件复制程序:
```c
#include <stdio.h>
#include <stdlib.h>
#define BUFFER_SIZE 1024
int main(int argc, char *argv[]) {
if (argc != 3) {
fprintf(stderr, "Usage: %s source_file dest_file\n", argv[0]);
exit(EXIT_FAILURE);
}
FILE *source_fp = fopen(argv[1], "rb");
if (source_fp == NULL) {
perror("fopen");
exit(EXIT_FAILURE);
}
FILE *dest_fp = fopen(argv[2], "wb");
if (dest_fp == NULL) {
perror("fopen");
exit(EXIT_FAILURE);
}
char buffer[BUFFER_SIZE];
size_t read_count, write_count;
while ((read_count = fread(buffer, 1, BUFFER_SIZE, source_fp)) > 0) {
write_count = fwrite(buffer, 1, read_count, dest_fp);
if (write_count != read_count) {
perror("fwrite");
exit(EXIT_FAILURE);
}
}
if (ferror(source_fp)) {
perror("fread");
exit(EXIT_FAILURE);
}
if (fclose(source_fp) != 0) {
perror("fclose");
exit(EXIT_FAILURE);
}
if (fclose(dest_fp) != 0) {
perror("fclose");
exit(EXIT_FAILURE);
}
return 0;
}
```
阅读全文
相关推荐
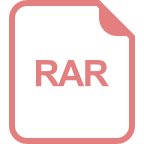






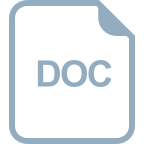
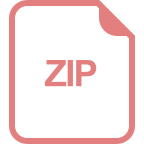
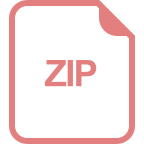