用C++代码写一个医院信息管理系统
时间: 2023-12-06 10:44:54 浏览: 293
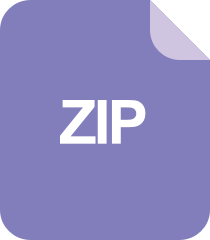
医院管理系统——MFC课程设计.zip
这个需求比较复杂,需要考虑很多方面。以下是一个简单的示例代码,实现基本的医院信息管理系统功能:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
// 定义病人结构体
struct Patient {
string name;
int age;
string gender;
string diagnosis;
};
// 定义医生结构体
struct Doctor {
string name;
string department;
};
// 定义医院类
class Hospital {
private:
vector<Patient> patients; // 病人列表
vector<Doctor> doctors; // 医生列表
public:
void addPatient(string name, int age, string gender, string diagnosis) {
Patient patient;
patient.name = name;
patient.age = age;
patient.gender = gender;
patient.diagnosis = diagnosis;
patients.push_back(patient);
}
void addDoctor(string name, string department) {
Doctor doctor;
doctor.name = name;
doctor.department = department;
doctors.push_back(doctor);
}
void listPatients() {
cout << "病人列表:" << endl;
for (int i = 0; i < patients.size(); i++) {
Patient patient = patients[i];
cout << "姓名:" << patient.name << " 年龄:" << patient.age << " 性别:" << patient.gender << " 诊断:" << patient.diagnosis << endl;
}
}
void listDoctors() {
cout << "医生列表:" << endl;
for (int i = 0; i < doctors.size(); i++) {
Doctor doctor = doctors[i];
cout << "姓名:" << doctor.name << " 科室:" << doctor.department << endl;
}
}
};
int main() {
Hospital hospital;
hospital.addPatient("张三", 30, "男", "感冒");
hospital.addPatient("李四", 40, "女", "胃炎");
hospital.addDoctor("王医生", "内科");
hospital.addDoctor("李医生", "外科");
hospital.listPatients();
hospital.listDoctors();
return 0;
}
```
这个代码实现了基本的医院信息管理系统,包括添加病人、添加医生、列出病人列表、列出医生列表等功能。当然,这只是一个简单的示例,实际的医院信息管理系统要考虑更多的因素,比如病人和医生的详细信息、医生的排班、病房管理等等。
阅读全文
相关推荐
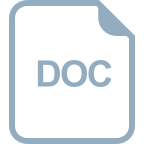
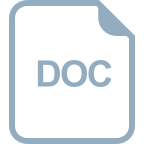
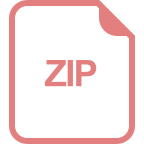
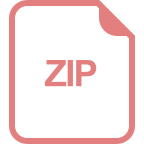
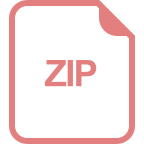
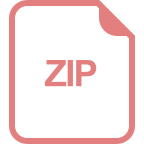
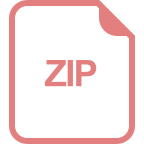
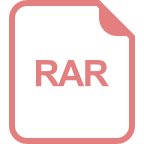
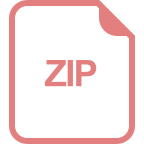
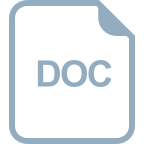
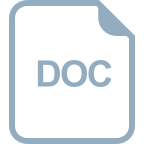
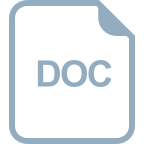
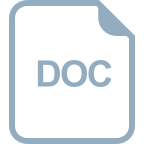
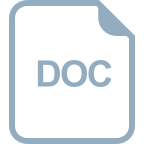
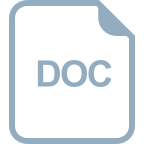
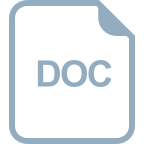
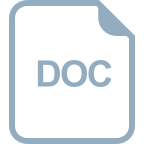
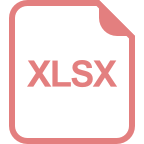
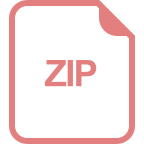