#include <stdio.h> struct student { int num; char name[20]; int score; }; struct student stud[10]; int main(void) { int i, sum = 0 ; for(i = 0; i < 10; i++){ scanf("%d%s%d", &stud[i].num, , &stud[i].score); sum += stud[i].score; } printf("aver=%d\n", sum/10); return 0; }
时间: 2023-11-27 10:50:34 浏览: 265
这段代码存在一些问题,主要是在 scanf 函数中缺少了一个参数。正确的代码如下:
```c
#include <stdio.h>
struct student {
int num;
char name[20];
int score;
};
struct student stud[10];
int main(void) {
int i, sum = 0 ;
for(i = 0; i < 10; i++) {
scanf("%d%s%d", &stud[i].num, stud[i].name, &stud[i].score); // 缺少了一个参数
sum += stud[i].score;
}
printf("aver=%d\n", sum/10);
return 0;
}
```
需要注意的是,该代码中只定义了一个长度为 20 的 char 数组来存储学生姓名,可能无法满足较长的姓名,需要根据实际情况进行修改。
相关问题
完成下列程序,该程序计算10名学生的平均成绩 输入答案 。 #include<stdio.h> #include<string.h> struct student { int num; char name[20]; int score; }; struct student stud[10]; int main(void) { int i,sum=0; for(i=0;i<10;i++) { scanf("%d%s%d",&stud[i].num, ,&stud[i].score); sum+=stud[i].score; } printf("aver=%d\n",sum/10); return 0; }
下面是完成后的程序:
```c
#include <stdio.h>
#include <string.h>
struct student {
int num;
char name[20];
int score;
};
struct student stud[10];
int main(void) {
int i, sum = 0;
for (i = 0; i < 10; i++) {
scanf("%d%s%d", &stud[i].num, stud[i].name, &stud[i].score);
sum += stud[i].score;
}
printf("aver=%d\n", sum / 10);
return 0;
}
```
程序中定义了一个结构体类型`student`,包含学号、姓名和成绩三个成员变量。在`main`函数中定义了一个包含10个`student`类型元素的数组`stud`,用于存储10个学生的信息。程序首先通过循环依次读入10个学生的信息,并将学生的成绩累加到变量`sum`中。最后输出`sum`除以10的结果,即10名学生的平均成绩。注意,`scanf`函数中的格式串应当为`"%d%s%d"`,其中`%s`对应字符串类型的姓名。
检查以下代码 进行纠错#include <stdio.h> struct student { int num; char name[20]; int score; }; struct student stud[10]; int main(void) { int i, sum = 0 ; for(i = 0; i < 10; i++){ scanf("%d%s%d", &stud[i].num, &stud[i].name , &stud[i].score); sum += stud[i].score; } printf("aver=%d\n", sum/10); return 0; }
这段代码有一个小错误,即在 scanf 函数中,第二个参数应该是 &stud[i].name,而不是 &stud[i].name[20]。因为 name 是一个字符数组,数组名本身就代表数组首元素的地址,不需要加上索引值。修改后的代码如下:
```c
#include <stdio.h>
struct student {
int num;
char name[20];
int score;
};
struct student stud[10];
int main(void) {
int i, sum = 0 ;
for(i = 0; i < 10; i++){
scanf("%d%s%d", &stud[i].num, stud[i].name , &stud[i].score);
sum += stud[i].score;
}
printf("aver=%d\n", sum/10);
return 0;
}
```
这样就可以正常运行了。
相关推荐
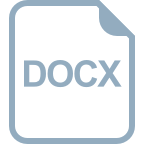
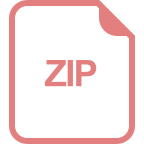
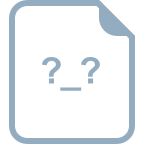
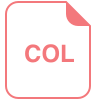
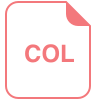










