vue2.0项目中自定义全局弹框
时间: 2023-12-11 12:05:27 浏览: 36
在 Vue 2.0 项目中,可以通过自定义全局弹框来实现对话框、提示框等弹框的统一管理。实现方式如下:
1. 创建一个 Vue 组件作为全局弹框的模板,包含弹框的 HTML 结构和样式,以及控制弹框显示隐藏的逻辑。
2. 在 main.js 中创建一个 Vue 实例,作为全局事件总线,用于在组件间通信。
3. 在该 Vue 实例中注册一个自定义指令,用于在组件中调用弹框。
4. 在组件中使用自定义指令,触发全局事件,传递弹框的参数给全局事件总线。
5. 在全局事件总线的相应方法中,根据传递的参数控制弹框的显示隐藏。
示例代码如下:
1. 弹框组件模板
```
<template>
<div v-show="visible" class="dialog">
<slot></slot>
</div>
</template>
<script>
export default {
data() {
return {
visible: false
};
},
methods: {
show() {
this.visible = true;
},
hide() {
this.visible = false;
}
}
};
</script>
<style>
.dialog {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: #fff;
padding: 20px;
border-radius: 5px;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.5);
z-index: 9999;
}
</style>
```
2. 全局事件总线
```
import Vue from 'vue';
export const eventBus = new Vue();
```
3. 自定义指令
```
import { eventBus } from './eventBus';
Vue.directive('dialog', {
bind(el, binding) {
el.addEventListener('click', () => {
eventBus.$emit('showDialog', binding.value);
});
}
});
```
4. 组件中调用弹框
```
<template>
<button v-dialog="{ title: 'Hello', content: 'World' }">Show Dialog</button>
</template>
```
5. 全局事件总线中控制弹框的显示隐藏
```
import Dialog from './Dialog.vue';
import { eventBus } from './eventBus';
new Vue({
render: h => h(App),
created() {
eventBus.$on('showDialog', ({ title, content }) => {
const dialog = new Vue({
render: h => h(Dialog, { props: { title, content } })
}).$mount();
document.body.appendChild(dialog.$el);
dialog.$children[0].show();
});
}
}).$mount('#app');
```
这样就实现了一个简单的全局弹框组件,可以在项目中任意组件间使用。
相关推荐
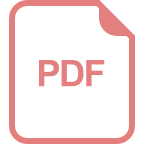
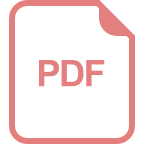
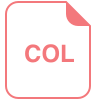
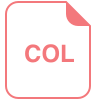
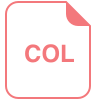
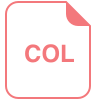
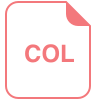









