用c++实现torchscript权重预加载
时间: 2023-04-10 09:01:17 浏览: 174
可以回答这个问题。可以使用以下代码实现torchscript权重预加载:
```c
#include <torch/script.h>
int main() {
// 加载模型
torch::jit::script::Module module;
try {
module = torch::jit::load("model.pt");
}
catch (const c10::Error& e) {
std::cerr << "error loading the model\n";
return -1;
}
// 加载权重
try {
module.load_state_dict(torch::load("weights.pt"));
}
catch (const c10::Error& e) {
std::cerr << "error loading the weights\n";
return -1;
}
// 使用模型进行推理
// ...
return 0;
}
```
相关问题
opencv+yolov5+c++安装
安装 OpenCV:
1. 在 Ubuntu 上执行以下命令安装依赖项:
```
sudo apt-get update
sudo apt-get install build-essential cmake git libgtk2.0-dev pkg-config libavcodec-dev libavformat-dev libswscale-dev
```
2. 下载 OpenCV 源代码:
```
git clone https://github.com/opencv/opencv.git
cd opencv
git checkout 4.5.1
```
3. 创建并进入 build 目录:
```
cd ..
mkdir build
cd build
```
4. 构建 OpenCV:
```
cmake -D CMAKE_BUILD_TYPE=RELEASE \
-D CMAKE_INSTALL_PREFIX=/usr/local \
-D WITH_CUDA=OFF \
-D BUILD_opencv_cudacodec=OFF \
-D WITH_LIBV4L=ON \
-D WITH_V4L=ON \
-D WITH_OPENGL=ON ..
make -j8
sudo make install
```
安装 YOLOv5:
1. 在终端执行以下命令:
```
git clone https://github.com/ultralytics/yolov5.git
cd yolov5
```
2. 安装依赖项:
```
pip install -r requirements.txt
```
3. 下载预训练权重:
```
wget https://github.com/ultralytics/yolov5/releases/download/v5.0/yolov5s.pt
```
4. 运行 YOLOv5:
```
python detect.py --weights yolov5s.pt --img 640 --conf 0.4 --source 0
```
其中,--weights 参数指定预训练权重的路径,--img 参数指定输入图像的大小,--conf 参数指定置信度阈值,--source 参数指定输入源(例如摄像头或视频文件)。
安装 C++ API:
1. 在 yolov5 目录下创建 build 目录并进入:
```
mkdir build
cd build
```
2. 运行 cmake 命令:
```
cmake ..
```
3. 运行 make 命令:
```
make
```
4. 安装生成的库文件:
```
sudo make install
```
5. 在 C++ 代码中使用 YOLOv5:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
#include <torch/script.h>
int main(int argc, const char* argv[]) {
// 加载模型
torch::jit::script::Module module = torch::jit::load("yolov5s.torchscript.pt");
module.eval();
// 读取图片
cv::Mat img = cv::imread("test.jpg");
// 转换图片格式
cv::cvtColor(img, img, cv::COLOR_BGR2RGB);
cv::Mat img_float;
img.convertTo(img_float, CV_32F, 1.0 / 255);
// 创建 Tensor
torch::Tensor tensor_image = torch::from_blob(img_float.data, {1, img.rows, img.cols, 3}, torch::kFloat32).permute({0, 3, 1, 2});
// 推理
std::vector<torch::jit::IValue> inputs;
inputs.push_back(tensor_image);
at::Tensor output = module.forward(inputs).toTensor();
// 处理输出
auto boxes = output.select(1, 0);
auto scores = output.select(1, 4);
auto labels = output.select(1, 5);
for (int i = 0; i < boxes.size(0); i++) {
float score = scores[i].item().toFloat();
if (score > 0.5) {
int label = labels[i].item().toInt();
float xmin = boxes[i][0].item().toFloat() * img.cols;
float ymin = boxes[i][1].item().toFloat() * img.rows;
float xmax = boxes[i][2].item().toFloat() * img.cols;
float ymax = boxes[i][3].item().toFloat() * img.rows;
cv::rectangle(img, cv::Point(xmin, ymin), cv::Point(xmax, ymax), cv::Scalar(0, 0, 255), 2);
}
}
// 显示结果
cv::imshow("result", img);
cv::waitKey();
return 0;
}
```
其中,"yolov5s.torchscript.pt" 是通过 torch.jit.script 函数导出的 TorchScript 模型文件,test.jpg 是输入图片文件。
阅读全文
相关推荐
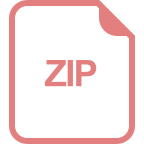
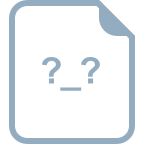
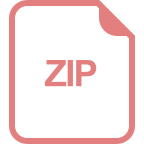
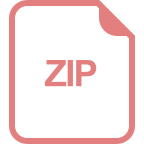
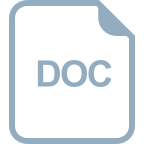
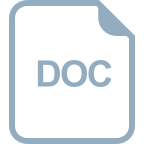
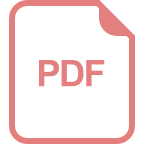
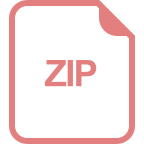
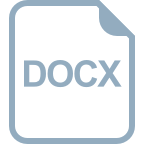
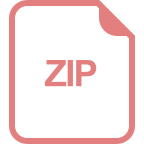
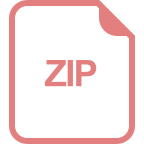