对于已经提取出来的三维根系图片,为二维序列图像450张,如何计算根系的总长度,利用python,举个详细的例子
时间: 2024-02-16 16:03:52 浏览: 30
计算根系的总长度可以通过以下步骤实现:
1. 安装必要的Python库
在Python中,我们可以使用NumPy和OpenCV库来处理图像和计算根系的总长度。首先,需要使用以下命令安装这些库:
```
pip install numpy opencv-python
```
2. 加载图像数据
我们可以使用OpenCV库中的`cv2.imread()`函数来加载图像数据。假设所有的图像数据都保存在一个名为`images`的文件夹中,我们可以使用以下代码来加载这些图像数据:
```python
import cv2
import os
images_folder = 'images'
images = []
for filename in os.listdir(images_folder):
img = cv2.imread(os.path.join(images_folder, filename))
images.append(img)
```
这个代码将会遍历`images`文件夹中的所有图像数据,并将它们存储在一个名为`images`的列表中。
3. 将图像转化为灰度图像
为了方便计算根系的长度,我们需要将彩色图像转化为灰度图像。这可以通过使用OpenCV库中的`cv2.cvtColor()`函数实现。
```python
gray_images = []
for img in images:
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
gray_images.append(gray_img)
```
这个代码将会遍历`images`列表中的所有图像数据,并将它们转化为灰度图像。转化后的灰度图像将会存储在一个名为`gray_images`的列表中。
4. 应用二值化算法
接下来,我们将会使用二值化算法将灰度图像转化为黑白图像。这可以通过使用OpenCV库中的`cv2.threshold()`函数实现。
```python
threshold_images = []
for gray_img in gray_images:
_, threshold_img = cv2.threshold(gray_img, 127, 255, cv2.THRESH_BINARY)
threshold_images.append(threshold_img)
```
这个代码将会遍历`gray_images`列表中的所有灰度图像,并将它们转化为黑白图像。转化后的黑白图像将会存储在一个名为`threshold_images`的列表中。
5. 应用形态学操作
为了去除图像中的噪点并连接相邻的根系像素,我们需要应用形态学操作。这可以通过使用OpenCV库中的`cv2.morphologyEx()`函数实现。
```python
morph_images = []
for threshold_img in threshold_images:
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
morph_img = cv2.morphologyEx(threshold_img, cv2.MORPH_OPEN, kernel)
morph_images.append(morph_img)
```
这个代码将会遍历`threshold_images`列表中的所有黑白图像,并将它们进行形态学操作。形态学操作后的图像将会存储在一个名为`morph_images`的列表中。
6. 计算根系的总长度
最后,我们可以计算所有根系的总长度。这可以通过遍历`morph_images`列表中的所有图像,并计算它们的像素数量实现。
```python
total_length = 0
for morph_img in morph_images:
total_length += cv2.countNonZero(morph_img)
```
这个代码将会遍历`morph_images`列表中的所有图像,并计算它们的像素数量。所有根系的总长度将会存储在一个名为`total_length`的变量中。
综上所述,以下是一个完整的计算根系总长度的Python代码:
```python
import cv2
import os
images_folder = 'images'
images = []
for filename in os.listdir(images_folder):
img = cv2.imread(os.path.join(images_folder, filename))
images.append(img)
gray_images = []
for img in images:
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
gray_images.append(gray_img)
threshold_images = []
for gray_img in gray_images:
_, threshold_img = cv2.threshold(gray_img, 127, 255, cv2.THRESH_BINARY)
threshold_images.append(threshold_img)
morph_images = []
for threshold_img in threshold_images:
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
morph_img = cv2.morphologyEx(threshold_img, cv2.MORPH_OPEN, kernel)
morph_images.append(morph_img)
total_length = 0
for morph_img in morph_images:
total_length += cv2.countNonZero(morph_img)
print('Total length of roots:', total_length)
```
相关推荐
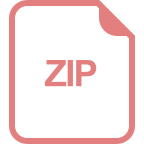
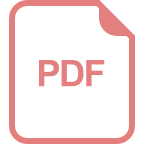
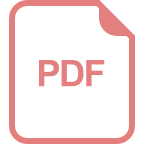








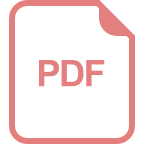
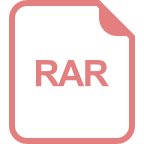
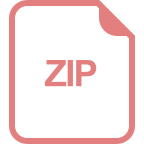
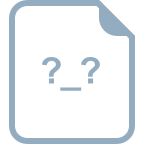