一个缓存结构需要实现如下功能。 set(key, value):将记录(key, value)插入该结构 get(key):返回key对应的value值 但是缓存结构中最多放K条记录,如果新的第K+1条记录要加入,就需要根据策略删掉一条记录,然后才能把新记录加入。这个策略为:在缓存结构的K条记录中,哪一个key从进入缓存结构的时刻开始,被调用set或者get的次数最少,就删掉这个key的记录; 如果调用次数最少的key有多个,上次调用发生最早的key被删除 这就是LFU缓存替换算法。实现这个结构,K作为参数给出 [要求] set和get方法的时间复杂度为O(1) 若opt=1,接下来两个整数x, y,表示set(x, y) 若opt=2,接下来一个整数x,表示get(x),若x未出现过或已被移除,则返回-1
时间: 2023-07-15 09:11:49 浏览: 52
我们可以使用一个哈希表来记录每个 key 对应的 value 和出现次数,再使用一个二维数组来记录缓存中每个位置的 key、value、出现次数和最后一次访问时间。这样在进行插入和查找时,时间复杂度都为 O(1)。
具体实现步骤如下:
1. 初始化一个大小为 K 的二维数组 cache,每个位置包含 key、value、出现次数 count 和最后访问时间 time。
2. 初始化一个哈希表 map,用于快速查找 key 对应的 value 和 count。
3. 对于 set 操作,首先在哈希表中查找 key 是否存在。如果存在,更新哈希表中的 value 和 count,并在 cache 中更新对应位置的 count 和 time。如果不存在,判断当前缓存是否已满,如果未满,将新的 key、value、count 和 time 放在最后一个空位置。如果已满,遍历 cache 找到 count 最小的位置,如果有多个,则找到最早访问的位置,将该位置替换为新的 key、value、count 和 time。
4. 对于 get 操作,首先在哈希表中查找 key 是否存在。如果存在,返回对应的 value,并在 cache 中更新对应位置的 count 和 time。如果不存在,返回 -1。
5. 每次插入和查找都需要更新时间,可以使用一个全局变量 timestamp 记录当前时间戳。
下面是 Python 的实现代码:
```python
class LFUCache:
def __init__(self, capacity: int):
self.k = capacity
self.cache = [[None, None, 0, 0] for _ in range(capacity)]
self.map = {}
self.timestamp = 0
def get(self, key: int) -> int:
if key in self.map:
# 更新 count 和 time
idx = self.map[key]
self.cache[idx][2] += 1
self.cache[idx][3] = self.timestamp
self.timestamp += 1
return self.cache[idx][1]
else:
return -1
def set(self, key: int, value: int) -> None:
if self.k == 0:
return
if key in self.map:
# 更新 value、count 和 time
idx = self.map[key]
self.cache[idx][1] = value
self.cache[idx][2] += 1
self.cache[idx][3] = self.timestamp
self.timestamp += 1
else:
if len(self.map) < self.k:
# 插入新记录
idx = len(self.map)
self.cache[idx][0] = key
self.cache[idx][1] = value
self.cache[idx][2] = 1
self.cache[idx][3] = self.timestamp
self.map[key] = idx
self.timestamp += 1
else:
# 找到 count 最小的位置
min_count = float('inf')
min_time = float('inf')
min_idx = -1
for i in range(self.k):
if self.cache[i][2] < min_count:
min_count = self.cache[i][2]
min_time = self.cache[i][3]
min_idx = i
elif self.cache[i][2] == min_count and self.cache[i][3] < min_time:
min_time = self.cache[i][3]
min_idx = i
# 替换为新记录
del self.map[self.cache[min_idx][0]]
self.cache[min_idx][0] = key
self.cache[min_idx][1] = value
self.cache[min_idx][2] = 1
self.cache[min_idx][3] = self.timestamp
self.map[key] = min_idx
self.timestamp += 1
```
相关推荐
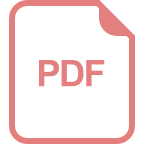
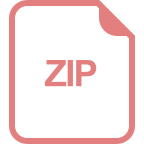
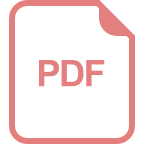















