def get(request): # 实例化SSHClient连接工具 ssh_client = paramiko.SSHClient() # 保存服务器密钥 ssh_client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) # 连接linux服务器 try: ssh_client.connect(hostname='192.168.233.128', port=22, username='root', password='12356789', timeout=2) except (EOFError, Exception) as e: request_data = {"code": 500, "message": "服务器连接失败", "data": []} return JsonResponse(request_data) # 返回了三个数据,第一个是输入命令,第2个是命今返回的结果,第3个是命令错误时返回的结果 # ps aux是linux服务器查询正在运行的所有应用程序的命令 stdin, stdout, stderr = ssh_client.exec_command('ps aux') # 读取返回结果,将编码转为utf8,避免乱码 df = stdout.read().decode('utf-8') # 创建一个临时文件存放命令返回的结果 # 因为linux服务器命令返回的数据是一个字符串,不能直接按空格截取,最好的方式就是写入文件读取一行再截取、拼接命令 # IO流, 数据存储到本地磁盘 filename = "tempdata.txt" # 打开文件,会在项目根目录打开,如果没有就会创建 w+为写入读取权限 fileObj = open(filename, mode='w+') # 将命令返回的结果写入文件 fileObj.write(df) # flush:保证数据成功写入文件。 fileObj.flush() fileObj.close()
时间: 2023-06-24 12:07:41 浏览: 76
这段代码是一个Django视图函数,实现了连接到一个Linux服务器并执行命令的功能。具体实现步骤如下:
1. 首先导入paramiko模块,该模块提供了SSH客户端的实现,可以用来连接远程Linux服务器。
2. 创建一个SSHClient对象,用来连接到Linux服务器,并设置自动添加新主机密钥的策略。
3. 使用connect()方法连接到指定的Linux服务器,需要传入服务器的IP地址、端口、用户名和密码等信息。
4. 如果连接失败,返回一个Json格式的错误信息。
5. 使用exec_command()方法执行命令,该方法返回三个对象:标准输入、标准输出和标准错误。在这里只需要标准输出对象。
6. 使用read()方法读取标准输出对象的内容,并将编码转换为UTF-8格式。
7. 创建一个临时文件,将命令返回的结果写入该文件。
8. 关闭文件并返回HttpResponse对象,该对象包含了命令返回的结果。
相关问题
import paramiko def shutdown_remote_system(): # 远程服务器的IP地址和SSH登录信息 remote_host = '192.168.0.106' remote_port = 22 remote_username = 'root' remote_password = '123' # 创建SSH连接 ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(remote_host, remote_port, remote_username, remote_password) # 执行关机命令 ssh.exec_command('sudo shutdown -h now') # 关闭SSH连接 ssh.close() 缺少是否已关机判断
你的代码是远程执行关机命令的代码,但是确实缺少判断远程系统是否已经关机的部分。
你可以通过在远程服务器上执行一个命令,判断系统是否已经关机。例如,你可以通过 ping 命令测试远程服务器是否可达。
以下是一个示例代码,可以在远程服务器上执行指定的命令并判断系统是否已经关机:
```python
import paramiko
import time
def shutdown_remote_system():
# 远程服务器的IP地址和SSH登录信息
remote_host = '192.168.0.106'
remote_port = 22
remote_username = 'root'
remote_password = '123'
# 创建SSH连接
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(remote_host, remote_port, remote_username, remote_password)
# 执行关机命令,并等待一段时间
ssh.exec_command('sudo shutdown -h now')
time.sleep(10)
# 判断系统是否已经关机
while True:
response = os.system('ping -c 1 ' + remote_host)
if response == 0:
time.sleep(5)
else:
break
# 关闭SSH连接
ssh.close()
```
这段代码中,使用了 `os.system` 函数来执行 ping 命令,判断远程服务器是否可达。如果远程服务器不可达,则说明系统已经关机。循环检测远程服务器是否可达,直到远程服务器不可达时退出循环。
import paramiko,time,os def shutdown_remote_system(): # 远程服务器的IP地址和SSH登录信息 remote_host = '192.168.0.106' remote_port = 22 remote_username = 'root' remote_password = '123' # 创建SSH连接 ssh = paramiko.SSHClient() ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy()) ssh.connect(remote_host, remote_port, remote_username, remote_password) # 执行关机命令 ssh.exec_command('sudo shutdown -h now') time.sleep(10) #判断系统是否已经关机 while True: response=os.system('ping -c 1'+remote_host) if response ==0: time.sleep(5) else: break # 关闭SSH连接 ssh.close() 判断中能否弹出提示:已关机
在这段代码中,判断系统是否已经关机的部分只是通过 ping 命令来判断主机是否可以 ping 通,如果可以 ping 通则返回 0,否则返回其他值。因此,如果系统已经关机,ping 命令会无法执行,不会返回任何值,也就没有办法弹出提示。如果您想要在系统已经关机后弹出提示,可以在 while 循环中添加一个判断条件,例如判断主机是否可以 ping 通,如果无法 ping 通则说明系统已经关机,可以弹出提示。具体实现方式可以参考下面的代码:
```
import paramiko,time,os
import tkinter.messagebox
def shutdown_remote_system():
# 远程服务器的IP地址和SSH登录信息
remote_host = '192.168.0.106'
remote_port = 22
remote_username = 'root'
remote_password = '123'
# 创建SSH连接
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(remote_host, remote_port, remote_username, remote_password)
# 执行关机命令
ssh.exec_command('sudo shutdown -h now')
time.sleep(10)
#判断系统是否已经关机
while True:
response = os.system('ping -c 1 '+remote_host)
if response == 0:
time.sleep(5)
else:
tkinter.messagebox.showinfo('提示', '已关机')
break
# 关闭SSH连接
ssh.close()
```
在这段代码中,我们使用了 Python 自带的 tkinter 模块中的 messagebox 子模块来弹出提示框,如果您的系统中没有安装 tkinter 模块,可以使用以下命令来安装:
```
pip install tkinter
```
相关推荐
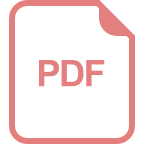
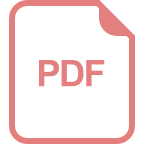
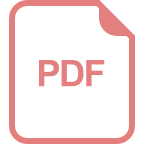












